Atlas插件Bundle间的通信方式RemoteTransactor、RemoteView、RemoteFragment
Atlas提供了3种Bundle与Bundle之间的通信方式:RemoteTransactor、RemoteView、RemoteFragment。RemoteTransactor是RemoteView、RemoteFragment通讯方式的简化版,仅仅为了Bundle和Bundle之间的通讯而存在。
以firstbundle调用secondbundle为例
RemoteView
在secondbundle中定义View实现IRemote
MyView.java
package cn.appblog.secondbundle;
import android.content.Context;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.widget.TextView;
public class MyView extends TextView {
public MyView(Context context) {
super(context);
}
public MyView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
public MyView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
}
MyFrameLayout.java
package cn.appblog.secondbundle;
import android.app.ProgressDialog;
import android.content.Context;
import android.os.Bundle;
import android.support.annotation.AttrRes;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.annotation.StyleRes;
import android.taobao.atlas.remote.IRemote;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.FrameLayout;
public class MyFrameLayout extends FrameLayout implements IRemote {
public MyFrameLayout(@NonNull Context context) {
super(context);
init();
}
public MyFrameLayout(@NonNull Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init();
}
public MyFrameLayout(@NonNull Context context, @Nullable AttributeSet attrs, @AttrRes int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
public void init() {
LayoutInflater inflater = LayoutInflater.from(getContext());
inflater.inflate(R.layout.layout_test, this);
this.findViewById(R.id.home_page).setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
ProgressDialog dialog = new ProgressDialog(getContext());
dialog.show();
}
});
}
@Override
public Bundle call(String commandName, Bundle args, IResponse callback) {
return null;
}
@Override
public <T> T getRemoteInterface(Class<T> interfaceClass, Bundle args) {
return null;
}
}
layout_test.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<cn.appblog.secondbundle.MyView
android:id="@+id/home_page"
android:layout_width="100dp"
android:layout_height="100dp"
android:textSize="18sp"
android:text="http://www.appblog.cn"
/>
</LinearLayout>
在secondbundle中注册View
在secondbundle的AndroidManifest.xml中注册MyFrameLayout(application标签下)
<meta-data android:name="atlas.view.intent.action.SECOND_VIEW" android:value="cn.appblog.secondbundle.MyFrameLayout"/>
在firstbundle中调用View
RemoteFactory.requestRemote(RemoteView.class, MainActivity.this, new Intent("atlas.view.intent.action.SECOND_VIEW"),
new RemoteFactory.OnRemoteStateListener<RemoteView>() {
@Override
public void onRemotePrepared(RemoteView iRemoteContext) {
FrameLayout layout = (FrameLayout) findViewById(R.id.layout_content);
FrameLayout.LayoutParams params = new FrameLayout.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT);
layout.addView(iRemoteContext, params);
}
@Override
public void onFailed(String s) {
Log.e("yezhou", s);
}
});
RemoteFragment
在secondbundle中定义Fragment实现IRemote
package cn.appblog.secondbundle;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.taobao.atlas.remote.IRemote;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class MyFragment extends Fragment implements IRemote {
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View v = inflater.inflate(R.layout.layout_test, container, false);
return v;
}
@Override
public Bundle call(String s, Bundle bundle, IResponse iResponse) {
return null;
}
@Override
public <T> T getRemoteInterface(Class<T> aClass, Bundle bundle) {
return null;
}
}
在secondbundle中注册View
在secondbundle的AndroidManifest.xml中注册MyFragment(application标签下)
<meta-data android:name="atlas.fragment.intent.action.SECOND_FRAGMENT" android:value="cn.appblog.secondbundle.MyFragment"/>
在firstbundle中调用View
RemoteFactory.requestRemote(RemoteFragment.class, MainActivity.this, new Intent("atlas.fragment.intent.action.SECOND_FRAGMENT"),
new RemoteFactory.OnRemoteStateListener<RemoteFragment>() {
@Override
public void onRemotePrepared(RemoteFragment iRemote) {
getSupportFragmentManager().beginTransaction().add(R.id.layout_fragment_content, iRemote).commit();
}
@Override
public void onFailed(String s) {
Log.e("yezhou", s);
}
});
RemoteTransactor
在公共module中定义接口
package cn.appblog.middleware;
public interface ICaculator {
int sum(int a,int b);
}
在secondbundle中定义IRemote实现类
package cn.appblog.secondbundle;
import android.content.ComponentCallbacks2;
import android.content.ContentProvider;
import android.content.res.Configuration;
import android.os.Bundle;
import android.taobao.atlas.remote.HostTransactor;
import android.taobao.atlas.remote.IRemote;
import android.taobao.atlas.runtime.ActivityLifeCycleObserver;
import android.taobao.atlas.runtime.RuntimeVariables;
import cn.appblog.middleware.ICaculator;
public class CaculatorRemote implements IRemote {
public CaculatorRemote() {
RuntimeVariables.androidApplication.registerComponentCallbacks(new ComponentCallbacks2() {
@Override
public void onTrimMemory(int level) {
if (level == ContentProvider.TRIM_MEMORY_UI_HIDDEN) {
HostTransactor remote = HostTransactor.get(CaculatorRemote.this);
if (remote != null) {
remote.call("SLEEP_NOTIFY", null, null);
}
}
}
@Override
public void onConfigurationChanged(Configuration newConfig) {
}
@Override
public void onLowMemory() {
}
});
}
@Override
public Bundle call(String s, Bundle bundle, IResponse iResponse) {
if (s.equalsIgnoreCase("sum")) {
int a = bundle.getInt("num1");
int b = bundle.getInt("num2");
bundle.putInt("result", a + b);
return bundle;
}
return null;
}
@Override
public <T> T getRemoteInterface(Class<T> aClass, Bundle bundle) {
if (aClass == ICaculator.class) {
T instance = (T) new ICaculator() {
@Override
public int sum(int a, int b) {
return a + b;
}
};
return instance;
}
return null;
}
}
在secondbundle中注册IRemote
在secondbundle的AndroidManifest.xml中注册MyFragment(application标签下)
<meta-data android:name="atlas.transaction.intent.action.SECOND_TRANSACTION" android:value="cn.appblog.secondbundle.CaculatorRemote"/>
在firstbundle中调用View
RemoteFactory.requestRemote(RemoteTransactor.class, MainActivity.this, new Intent("atlas.transaction.intent.action.SECOND_TRANSACTION"),
new RemoteFactory.OnRemoteStateListener<RemoteTransactor>() {
@Override
public void onRemotePrepared(RemoteTransactor iRemote) {
// if you want remote call you
iRemote.registerHostTransactor(new IRemote() {
@Override
public Bundle call(String s, Bundle bundle, IResponse iResponse) {
Toast.makeText(RuntimeVariables.androidApplication, "Command is " + s, Toast.LENGTH_SHORT).show();
return null;
}
@Override
public <T> T getRemoteInterface(Class<T> aClass, Bundle bundle) {
return null;
}
});
ICaculator caculator = iRemote.getRemoteInterface(ICaculator.class, null);
Toast.makeText(RuntimeVariables.androidApplication, "1+1 = " + caculator.sum(1, 1), Toast.LENGTH_SHORT).show();
//you can also use this
// Bundle bundle = new Bundle();
// bundle.putInt("num1", 1);
// bundle.putInt("num2", 1);
// Bundle result = iRemote.call("sum", bundle, null);
// Toast.makeText(RuntimeVariables.androidApplication, "1+1 = " + result.getInt("result"), Toast.LENGTH_SHORT).show();
}
@Override
public void onFailed(String s) {
Log.e("UserRemoteActivity", s);
}
});
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/18/communication-methods-between-atlas-plugin-bundles-remotetransactor-remoteview-and-remotefragment/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

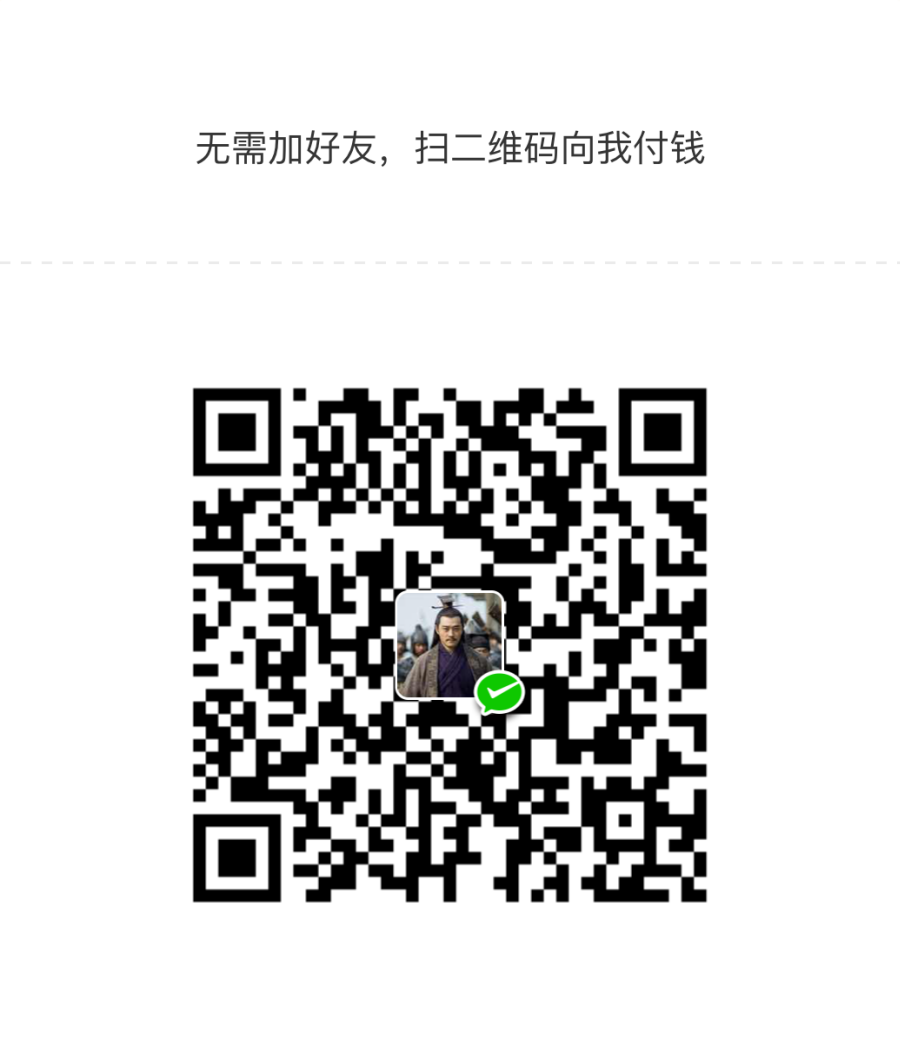

共有 0 条评论