Python搭建HTTP服务器:API接口(支持RESTful API)
API接口包含:
- GET API
- POST API
- RESTful API
- 文件上传API
# coding=utf-8
import os
from flask import Flask, jsonify, request, redirect, url_for
from werkzeug import secure_filename
UPLOAD_FOLDER = 'D:/www/upload'
ALLOWED_EXTENSIONS = set(['txt', 'apk', 'zip', 'rar'])
app = Flask(__name__)
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
# Hello World
@app.route('/')
def hello_world():
return 'Hello World!'
# RESTful API
@app.route("/user/<int:id>")
def user(id):
return jsonify(id=id, username='Joe.Ye', head_url='http://www.androidios.cc/images/avatar.png')
# GET API
@app.route("/user/info")
def user_info():
id = request.args.get("id")
print request.headers
return jsonify(id=id, username='Joe.Ye', head_url='http://www.androidios.cc/images/avatar.png')
# POST API
@app.route("/login", methods=["POST"])
def login():
username = request.form.get("username");
password = request.form.get("password");
if username=='AppBlog.CN' and password=='123456':
return jsonify(code=1, message=u'登录成功')
return jsonify(code=0, message=u'用户名或者密码错误')
# POST API(JSON)
@app.route("/login/json", methods=["POST"])
def login_json():
json = request.get_json();
username = json.get("username");
password = json.get("password");
if username=='AppBlog.CN' and password=='123456':
return jsonify(code=1, message=u'登录成功')
return jsonify(code=0, message=u'用户名或者密码错误')
# POST API
@app.route("/user/new", methods=["POST"])
def user_create():
json = request.get_json()
print json
id = json.get("id")
username = json.get("username");
print id
print username
return jsonify(code=1, message=u"保存用户成功")
# POST API
@app.route("/user/edit", methods=["POST"])
def user_edit():
id = request.form.get("id")
username = request.form.get("username")
print request.headers
return jsonify(code=1, message=u"更新用户用户成功")
# 校验文件类型
def allowed_file(filename):
return '.' in filename and \
filename.rsplit('.', 1)[1] in ALLOWED_EXTENSIONS
# 文件上传API,请查看文章底部附言
@app.route("/upload", methods=['GET', 'POST'])
def upload():
if request.method == 'POST':
username = request.form.get("username")
print 'username = ' + username
file = request.files['file']
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
print 'file = ' + filename
file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename))
#return redirect(url_for('upload'))
return jsonify(code=1, message=u'文件上传成功')
return """
<!doctype html>
<title>Upload new File</title>
<h1>Upload new File</h1>
<form action="" method="post" enctype="multipart/form-data">
<input type=file name=file>
<input type=submit value=Upload>
</form>
<p>%s</p>
""" % "<br>".join(os.listdir(app.config['UPLOAD_FOLDER'],))
if __name__ == '__main__':
app.run(host='0.0.0.0')
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/19/python-deploy-http-server-restful-api/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

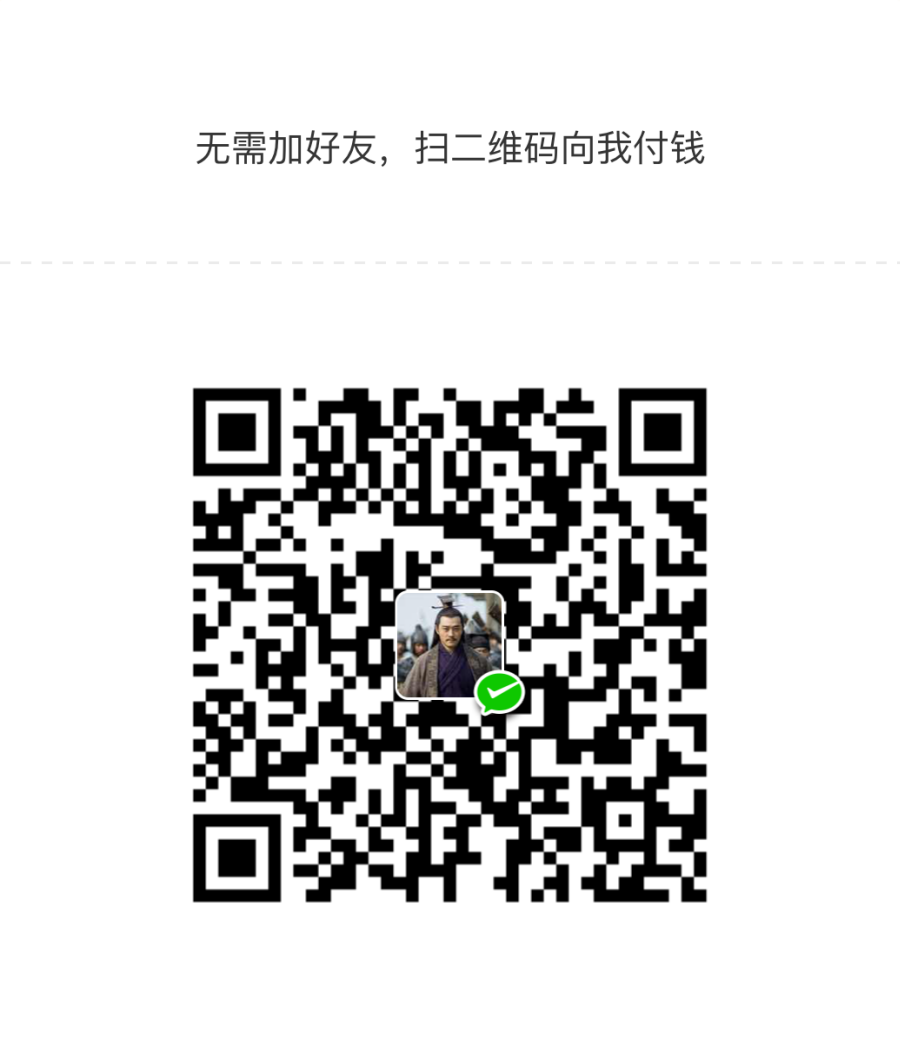
Python搭建HTTP服务器:API接口(支持RESTful API)
API接口包含:
GET API
POST API
RESTful API
文件上传API
# coding=utf-8
import os
from flask import Flask, jsonify, request, redirect, url_for
fro……

文章目录
关闭
共有 0 条评论