React Native学习之RN调用原生UI组件方法
实例1:原生控件,通过属性调用原生控件的方法,实现跑马灯效果
实例2:组合控件,通过属性调用组合控件的方法,实现选中状态效果
注:React Native中没有CheckBox组件
FocusedTextViewManager.java
public class FocusedTextViewManager extends SimpleViewManager<FocusedTextView> {
private ThemedReactContext context;
@Override
public String getName() {
return "FocusedTextView";
}
@Override
protected FocusedTextView createViewInstance(ThemedReactContext reactContext) {
context = reactContext;
FocusedTextView focusedTextView = new FocusedTextView(context);
focusedTextView.setText("欢迎观临 React Native APP 开发社区,一个靠谱的跨平台移动开发技术交流平台");
focusedTextView.setTextColor(Color.BLUE);
focusedTextView.setSingleLine(true);
focusedTextView.setTextSize(20);
focusedTextView.setEllipsize(TextUtils.TruncateAt.MARQUEE);
return focusedTextView;
}
//暴露属性
@ReactProp(name = "text")
public void setText(FocusedTextView view, String text){
view.setText(text);
}
}
SettingItemViewManager.java
public class SettingItemViewManager extends SimpleViewManager<SettingItemView> {
private ThemedReactContext context;
@Override
public String getName() {
return "SettingItemView";
}
@Override
protected SettingItemView createViewInstance(ThemedReactContext reactContext) {
context = reactContext;
SettingItemView itemView = new SettingItemView(context);
itemView.setDesc("自动更新已经关闭");
itemView.setTitle("设置是否自动更新");
return itemView;
}
@ReactProp(name = "desc")
public void setDesc(SettingItemView view, String desc){
view.setDesc(desc);
}
@ReactProp(name = "title")
public void setTitle(SettingItemView view, String title){
view.setTitle(title);
}
@ReactProp(name = "isChecked")
public void setChecked(SettingItemView view, boolean isChecked){
view.setChecked(isChecked);
}
}
MyNativeModule.java
public class MyNativeModule extends ReactContextBaseJavaModule implements ActivityEventListener, LifecycleEventListener {
private ReactApplicationContext context;
public static final int REQUEST_CODE = 1;
public MyNativeModule(ReactApplicationContext reactContext) {
super(reactContext);
context = reactContext;
}
@Override
public String getName() {
//一定要返回一个名字,在RN代码里面需要使用这个名字来调用该类的方法
return "MyNativeModule";
}
//函数不能有返回值,因为被调用的原生代码是异步的,原生代码执行结束之后只能通过回调函数或者发送消息给RN
//必须声明ReactMethod注解
@ReactMethod
public void showNativeMsg(String msg) {
Toast.makeText(context, msg, Toast.LENGTH_LONG).show();
}
@ReactMethod
public void startMyActivity(){
Intent intent = new Intent(context, MyActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
}
MyReactPackage.java
public class MyReactPackage implements ReactPackage {
@Override
public List<NativeModule> createNativeModules(ReactApplicationContext reactContext) {
return Arrays.<NativeModule>asList(
new MyNativeModule(reactContext)
);
}
@Override
public List<Class<? extends JavaScriptModule>> createJSModules() {
return Collections.emptyList();
}
@Override
public List<ViewManager> createViewManagers(ReactApplicationContext reactContext) {
return Arrays.<ViewManager>asList(
new FocusedTextViewManager(),
new SettingItemViewManager()
);
}
}
MainApplication.java
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(), new MyReactPackage()
);
}
FocusedTextView.js
import React, { Component, PropTypes } from 'react';
import {
requireNativeComponent,
Text,
View
} from 'react-native';
let iface = {
name:'FocusedTextView',
propTypes:{
text:PropTypes.string,
...View.propTypes
},
};
module.exports = requireNativeComponent('FocusedTextView', iface);
SettingItemView.js
import React, { Component, PropTypes } from 'react';
import {
requireNativeComponent,
Text,
View
} from 'react-native';
let iface = {
name:'SettingItemView',
propTypes:{
desc:PropTypes.string,
title:PropTypes.string,
isChecked:PropTypes.bool,
...View.propTypes
},
};
module.exports = requireNativeComponent('SettingItemView', iface);
index.android.js
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View,
NativeModules,
TouchableOpacity
} from 'react-native';
import FocusedTextView from './FocusedTextView.js';
import SettingItemView from './SettingItemView.js';
import Dimensions from 'Dimensions';
let width = Dimensions.get('window').width;
class RNAPP extends Component {
constructor(props) {
super(props);
this.state = {
isChecked:false,
desc:'自动更新已经关闭',
title:'设置是否自动更新',
};
}
render() {
return (
<View style={styles.container}>
<Text style={styles.welcome}>
Welcome to React Native!
</Text>
<Text style={styles.welcome}>
React Native混合原生开发
</Text>
<Text style={styles.instructions}>
Powered by rnapp.cc
</Text>
<Text onPress={this.startMyActivity.bind(this)} style={styles.btn}>启动原生界面</Text>
<FocusedTextView
style={{ width: width - 40, height: 30, marginTop: 10 }}
text='欢迎观临 React Native APP 开发社区,一个靠谱的跨平台移动开发技术交流平台'
/>
<TouchableOpacity onPress={this.setAutoUpdate}>
<SettingItemView
style={{ width: width - 20, height: 68, marginTop: 10 }}
title={this.state.title}
desc={this.state.desc}
isChecked={this.state.isChecked}
/>
</TouchableOpacity>
</View>
);
}
startMyActivity = () => {
NativeModules.MyNativeModule.startMyActivity();
}
setAutoUpdate = () => {
if(this.state.isChecked){
this.setState({
isChecked:false,
desc:'自动更新已经关闭',
});
} else {
this.setState({
isChecked:true,
desc:'自动更新开启啦',
});
}
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
btn: {
marginTop: 20,
marginLeft: 10,
marginRight: 10,
width: 260,
height: 35,
backgroundColor: '#3BC1FF',
color: '#fff',
lineHeight: 24,
fontWeight: 'bold',
textAlign: 'center',
textAlignVertical:'center'
},
});
AppRegistry.registerComponent('RNAPP', () => RNAPP);
MyActivity.java
public class MyActivity extends AppCompatActivity {
private SettingItemView siv_update;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
siv_update = (SettingItemView) this.findViewById(R.id.siv_update);
siv_update.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (siv_update.isChecked()) {
siv_update.setChecked(false);
siv_update.setDesc("自动更新已经关闭");
} else {
siv_update.setChecked(true);
siv_update.setDesc("自动更新开启啦!");
}
}
});
}
public void onBack(View v) {
finish();
}
}
SettingItemView.java
public class SettingItemView extends RelativeLayout {
private TextView tv_desc;
private TextView tv_title;
private CheckBox cb_status;
public SettingItemView(Context context) {
super(context);
iniView(context);
}
public SettingItemView(Context context, AttributeSet attrs) {
super(context, attrs);
iniView(context);
}
public SettingItemView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
iniView(context);
}
private void iniView(Context context) {
View.inflate(context, R.layout.setting_item_view, this);
cb_status = (CheckBox) this.findViewById(R.id.cb_status);
tv_desc = (TextView) this.findViewById(R.id.tv_desc);
tv_title = (TextView) this.findViewById(R.id.tv_title);
}
/**
* 校验组合控件是否选中
* @return
*/
public boolean isChecked() {
return cb_status.isChecked();
}
/**
* 设置组合控件的状态
* @param checked
*/
public void setChecked(boolean checked) {
cb_status.setChecked(checked);
}
/**
* 设置组合控件的描述信息
* @param text
*/
public void setDesc(String text) {
tv_desc.setText(text);
}
/**
* 设置组合控件的标题
* @param title
*/
public void setTitle(String title){
tv_title.setText(title);
}
}
activity_my.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
xmlns:android="http://schemas.android.com/apk/res/android"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="200dp"
android:text="Powered by RNAPP.CC"
android:paddingLeft="10dp"
android:paddingRight="10dp"
android:textSize="20sp"
android:layout_gravity="center_horizontal"/>
<Button
android:onClick="onBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="20dp"
android:background="#3BC1FF"
android:paddingLeft="10dp"
android:paddingRight="10dp"
android:text="原生按钮 - 点击退出"
android:textSize="16sp"
android:textColor="#FFFFFF"
/>
<cc.rnapp.view.FocusedTextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:text="欢迎观临 React Native APP 开发社区,一个靠谱的跨平台移动开发技术交流平台"
android:textSize="18sp"
android:textColor="#FF0000"
android:ellipsize="marquee"
android:singleLine="true"
/>
<cc.rnapp.view.SettingItemView
android:id="@+id/siv_update"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
/>
</LinearLayout>
setting_item_view.xml
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="60dp"
xmlns:android="http://schemas.android.com/apk/res/android"
>
<TextView
android:id="@+id/tv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:text="设置是否自动更新"
android:textColor="#000000"
android:textSize="20sp"/>
<TextView
android:id="@+id/tv_desc"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/tv_title"
android:layout_marginLeft="10dp"
android:text="自动更新已经关闭"
android:textColor="#88000000"
android:textSize="18sp"
/>
<CheckBox
android:id="@+id/cb_status"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_marginRight="10dp"
android:layout_centerVertical="true"
android:clickable="false"
android:focusable="false"
/>
<View
android:layout_width="match_parent"
android:layout_height="0.2dp"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:layout_alignParentBottom="true"
android:background="#000000"
/>
</RelativeLayout>
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/react-native-learning-rn-calling-native-ui-component-methods/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

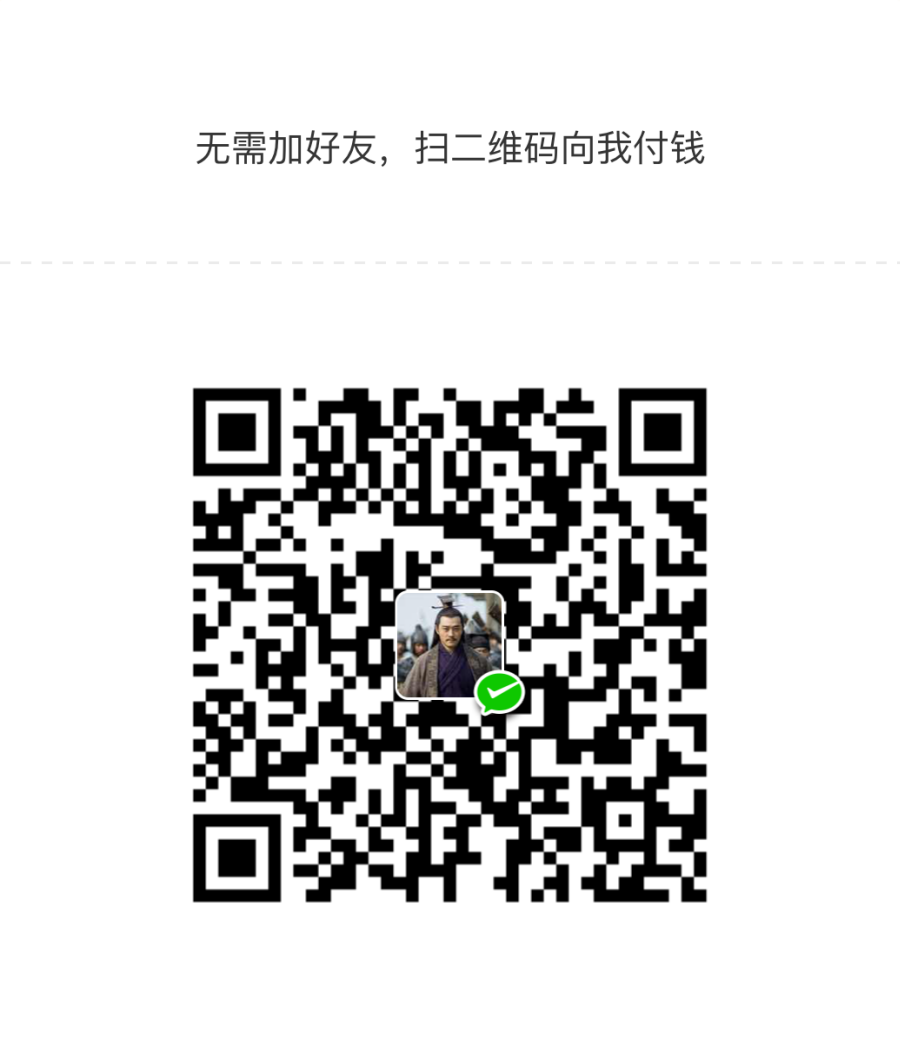

共有 0 条评论