React Native之微信登陆、分享与支付
React Native之微信登陆、分享与支付react-native-wechat
项目地址:https://github.com/weflex/react-native-wechat
准备
微信开放平台(https://open.weixin.qq.com/)
- 应用签名:06b0d7b419ad0bd12844689a2f1a36c1
- 包名:cn.appblog.mm
keystore和key的密码都是一样的
Android Studio 修改包名
React Native 初始化的默认包名 com.wechatdemo 与 微信开放平台应用的包名 一致的可以跳过此步骤。
(1)新建一个包名(也就是目标包名)
(2)将之前包下的所有文件拖到新建的包下,Android Studio 会自动修改文件中引入的包名。但是有些注释或者代码中文本形式的需要替换的,则需要手动修改,或者先删除掉否则是无法拖拽的。
(3)修改清单文件AndroidManifest.xml和build.gradle (applicationId) 中的包名
(4)Bulid Clean一下,提示找不到R文件
(5)修改 R文件的引入路径 以及 BuildConfig (MainApplication.java) 文件的引入路径,这两个文件都是自动生成的
安装
安装组件
npm install react-native-wechat --save
链接组件
// React Native v0.27+
react-native link react-native-wechat
// React Native v0.27-
rnpm link react-native-wechat
重新编译
react-native run-android
react-native run-ios
使用
(1)创建包 net.izengzhi.mmm.wxapi,并在包下创建类 WXEntryActivity,这是向微信发送请求和从微信接收响应所必需的。
package your.package.wxapi;
import android.app.Activity;
import android.os.Bundle;
import com.theweflex.react.WeChatModule;
public class WXEntryActivity extends Activity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
WeChatModule.handleIntent(getIntent());
finish();
}
}
(2)在 AndroidManifest.xml 清单文件中添加声明
<manifest>
<application>
<!-- 微信Activity -->
<activity
android:name=".wxapi.WXEntryActivity"
android:label="@string/app_name"
android:exported="true"
/>
</application>
</manifest>
(3)在 proguard-rules.pro 添加代码混淆规则
-keep class com.tencent.mm.sdk.** {
*;
}
实例演示
主要演示分享到好友/朋友圈的链接以及文本,关于更多的分享实例,例如文件、图片、视频、语音等等可以查看项目的说明文件即可。
分享实例步骤:
- 注册应用
- 本文/朋友圈分享
- Android测试应用需要Release打包测试
- iOS版本直接测试即可
源码:
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
TouchableOpacity,
View
} from 'react-native';
import * as WeChat from 'react-native-wechat';
//某个文件中有多个export的模块
//引入时统一归集到一个名为WeChat的变量名下
class Mmm extends Component {
constructor(props) {
super(props);
this.state = {
apiVersion: 'waiting...',
wxAppInstallUrl: 'waiting...',
isWXAppSupportApi: 'waiting...',
isWXAppInstalled: 'waiting...',
};
}
async componentDidMount() {
try {
await WeChat.registerApp('wx3c0fca268b5898d5');
this.setState({
apiVersion: await WeChat.getApiVersion(),
// wxAppInstallUrl: await WeChat.getWXAppInstallUrl(),
isWXAppSupportApi: await WeChat.isWXAppSupportApi(),
isWXAppInstalled: await WeChat.isWXAppInstalled()
});
console.log(this.state);
} catch (e) {
console.error(e);
}
console.log(WeChat);
// console.log('getApiVersion', typeof WeChat.getApiVersion);
// console.log('getWXAppInstallUrl', typeof WeChat.getWXAppInstallUrl);
// console.log('sendRequest', typeof WeChat.sendRequest);
// console.log('registerApp', typeof WeChat.registerApp);
// console.log('sendErrorCommonResponse', typeof WeChat.sendErrorCommonResponse);
// console.log('sendErrorUserCancelResponse', typeof WeChat.sendErrorUserCancelResponse);
// console.log('sendAuthRequest', typeof WeChat.sendAuthRequest);
// console.log('getWXAppInstallUrl', typeof WeChat.getWXAppInstallUrl);
// console.log('openWXApp', typeof WeChat.openWXApp);
// console.log('registerAppWithDescription', typeof WeChat.registerAppWithDescription);
// console.log('isWXAppSupportApi', typeof WeChat.isWXAppSupportApi);
// console.log('isWXAppInstalled', typeof WeChat.isWXAppInstalled);
}
render() {
return (
<View style={styles.container}>
<View style={styles.flex} />
<Text style={styles.text}>API版本:{this.state.apiVersion}</Text>
<Text style={styles.text}>微信注册URL:{this.state.wxAppInstallUrl}</Text>
<Text style={styles.text}>是否支持API:{String(this.state.isWXAppSupportApi) }</Text>
<Text style={styles.text}>是否安装微信:{String(this.state.isWXAppInstalled) }</Text>
<TouchableOpacity onPress={this._openWXApp}>
<Text style={styles.text}>打开微信</Text>
</TouchableOpacity>
<TouchableOpacity onPress={this._sendAuthRequest}>
<Text style={styles.text}>发送验证请求</Text>
</TouchableOpacity>
<TouchableOpacity onPress={this._shareToTimeline}>
<Text style={styles.text}>分享到微信朋友圈</Text>
</TouchableOpacity>
<TouchableOpacity onPress={this._shareToSession}>
<Text style={styles.text}>分享到微信好友或群</Text>
</TouchableOpacity>
<View style={styles.flex} />
<Text style={styles.author}>Powered by RNAPP.CC</Text>
</View>
);
}
async _openWXApp() {
await WeChat.openWXApp();
}
/*
async _sendAuthRequest() {
await WeChat.sendAuthRequest();
}
*/
async _shareToSession() {
let result =await WeChat.shareToSession({
title: '微信好友或群组分享测试(链接)',
description: 'React Native APP 开发社区 - 靠谱的 RN APP 和 Android/iOS 开发社区 RNAPP.CC',
thumbImage: 'http://www.ouloon.com/images/RNAPP.png',
type: 'news',
webpageUrl:'http://www.rnapp.cc'
});
console.log('分享到微信好友或群组是否成功:', result);
}
async _shareToTimeline() {
let result =await WeChat.shareToTimeline({
title: '微信朋友圈分享测试(链接)',
description: 'React Native APP 开发社区 - 靠谱的 RN APP 和 Android/iOS 开发社区 RNAPP.CC',
thumbImage: 'http://www.ouloon.com/images/RNAPP.png',
type: 'news',
webpageUrl:'http://www.rnapp.cc'
});
console.log('分享到微信朋友圈是否成功:', result);
}
/*
_shareToSession = () => {
WeChat.isWXAppInstalled()
.then((isInstalled) => {
console.log('微信是否已经安装:' + isInstalled);
if (isInstalled) {
WeChat.shareToSession({
title: '微信好友或群组分享测试(链接)',
description: 'React Native APP 开发社区 - 靠谱的 RN APP 和 Android/iOS 开发社区 RNAPP.CC',
thumbImage: 'http://www.ouloon.com/images/RNAPP.png',
type: 'news',
webpageUrl: 'http://www.rnapp.cc'
})
.catch((error) => {
alert(error.message);
});
} else {
alert('没有安装微信软件,请您安装微信之后再试');
}
});
}
*/
/*
_shareToTimeline = () => {
WeChat.isWXAppInstalled()
.then((isInstalled) => {
if (isInstalled) {
WeChat.shareToTimeline({
title: '微信朋友圈分享测试(链接)',
description: 'React Native APP 开发社区 - 靠谱的 RN APP 和 Android/iOS 开发社区 RNAPP.CC',
thumbImage: 'http://www.ouloon.com/images/RNAPP.png',
type: 'news',
webpageUrl: 'http://www.rnapp.cc'
})
.catch((error) => {
alert(error.message);
});
} else {
alert('没有安装微信软件,请您安装微信之后再试');
}
});
}
*/
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
text: {
fontSize: 20,
marginTop: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
flex: {
flex: 1,
},
author: {
justifyContent: 'flex-end',
alignItems: 'center',
marginBottom: 10,
textAlign: 'center',
fontSize: 16,
color: '#3BC1FF',
},
});
AppRegistry.registerComponent('WechatDemo', () => Mmm);
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/react-native-wechat-login-sharing-and-payment/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

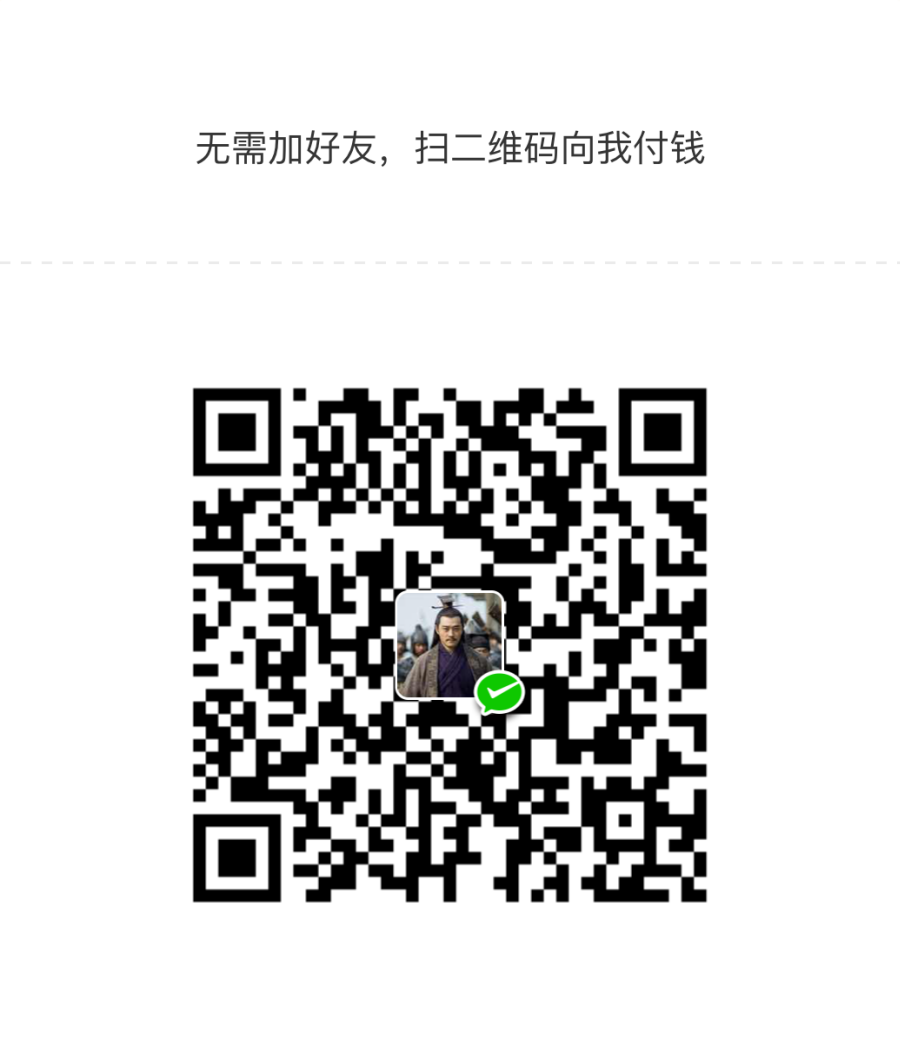

共有 0 条评论