Android NDK基础21:C++_继承_多态
继承
#include <iostream>
//代码的重用性
using namespace std;
//人类
class Human {
public:
void say() {
cout << "说话" << endl;
}
protected:
char* name;
int age;
};
//男人
class Man : public Human {
public:
//泡妞
void chasing(){
cout << "泡妞" << endl;
}
private:
//兄弟
char* brother;
};
void work(Human& h) {
h.say();
}
void main() {
Man m;
m.say();
//父类类型的引用或指针
Human* h_p = &m;
h_p->say();
//引用
Human &h1 = m;
h1.say();
//子类对象初始化父类类型的对象
Human h2 = m;
system("pause");
}
向父类构造方法传参
//向父类构造方法传参
//人类
class Human {
public:
Human(char* name, int age) {
this->name = name;
this->age = age;
}
void say() {
cout << "说话" << endl;
}
protected:
char* name;
int age;
};
//男人
class Man : public Human {
public:
//给父类构造函数传参,同时给属性对象赋值
Man(char *brother, char *s_name, int s_age, char *h_name, int h_age) : Human(s_name, s_age), h(h_name, h_age) {
this->brother = brother;
}
//泡妞
void chasing() {
cout << "泡妞" << endl;
}
private:
//兄弟
char* brother;
Human h;
};
void main() {
Man m("Joe", "ZhangSan", 18, "Lisi", 18);
system("pause");
}
构造函数与析构函数调用的顺序
//构造函数与析构函数调用的顺序
class Human {
public:
Human(char* name, int age) {
this->name = name;
this->age = age;
cout << "Human 构造函数" << endl;
}
~Human() {
cout << "Human 析构函数" << endl;
}
void say() {
cout << "说话" << endl;
}
protected:
char* name;
int age;
};
//男人
class Man : public Human {
public:
//给父类构造函数传参,同时给属性对象赋值
an(char *brother, char *s_name,int s_age) : Human(s_name, s_age) {
this->brother = brother;
cout << "Man 构造函数" << endl;
}
~Man() {
cout << "Man 析构函数" << endl;
}
//泡妞
void chasing() {
cout << "泡妞" << endl;
}
private:
//兄弟
char* brother;
};
void func() {
//父类的构造函数先调用
//子类的析构函数先调用
Man m("Joe", "Android", 18);
}
void main() {
func();
system("pause");
}
子类对象调用父类的成员
//子类对象调用父类的成员
class Human {
public:
Human(char* name, int age) {
this->name = name;
this->age = age;
cout << "Human 构造函数" << endl;
}
~Human() {
cout << "Human 析构函数" << endl;
}
void say() {
cout << "说话" << endl;
}
public:
char* name;
int age;
};
//男人
class Man : public Human {
public:
//给父类构造函数传参,同时给属性对象赋值
Man(char *brother, char *s_name, int s_age) : Human(s_name, s_age) {
this->brother = brother;
cout << "Man 构造函数" << endl;
}
~Man() {
cout << "Man 析构函数" << endl;
}
//泡妞
void chasing() {
cout << "泡妞" << endl;
}
void say() {
cout << "男人喜欢装逼" << endl;
}
private:
//兄弟
char* brother;
};
void main() {
//是覆盖,并非多态
Man m("Joe", "Android", 18);
m.say();
//Human h = m;
//h.say();
m.Human::say();
m.Human::age = 10;
system("pause");
}
多继承
//人
class Person {
};
//公民
class Citizen {
};
//学生,既是人,又是公民
class Student : public Person, public Citizen {
};
继承的访问修饰
//继承的访问修饰
//基类中 继承方式 子类中
//public & public继承 = > public
//public & protected继承 = > protected
//public & private继承 = > private
//
//protected & public继承 = > protected
//protected & protected继承 = > protected
//protected & private继承 = > private
//
//private & public继承 = > 子类无权访问
//private & protected继承 = > 子类无权访问
//private & private继承 = > 子类无权访问
//人类
class Human {
public:
void say() {
cout << "说话" << endl;
}
private:
char* name;
int age;
};
//男人
class Man : protected Human {
public:
//泡妞
void chasing() {
cout << "泡妞" << endl;
}
private:
//兄弟
char* brother;
};
继承的二义性
//继承的二义性
//虚继承,不同路径继承来的同名成员只有一份拷贝,解决不明确的问题
class A {
public:
char* name;
};
class A1 : virtual public A {
};
class A2 : virtual public A {
};
class B : public A1, public A2 {
};
void main() {
B b;
b.name = "Joe";
//指定父类显示调用
//b.A1::name = "Joe";
//b.A2::name = "Joe";
system("pause");
}
多态
虚函数
//多态(程序的扩展性)
//动态多态:程序运行过程中,觉得哪一个函数被调用(重写)
//静态多态:重载
//发生动态多态的条件:
//1.继承
//2.父类的引用或者指针指向子类的对象
//3.函数的重写
#include "Plane.h"
#include "Jet.h"
#include "Copter.h"
//业务函数
void bizPlay(Plane& p) {
p.fly();
p.land();
}
void main() {
//普通飞机
Plane p1;
bizPlay(p1);
//喷气式飞机
Jet p2;
bizPlay(p2);
//直升飞机
Copter p3;
bizPlay(p3);
system("pause");
}
Plane.h
#pragma once
//普通飞机
class Plane {
public:
virtual void fly(); //只有声明virtual函数才能产生多态
virtual void land();
};
Plane.cpp
#include "Plane.h"
#include <iostream>
using namespace std;
void Plane::fly() {
cout << "起飞" << endl;
}
void Plane::land() {
cout << "着陆" << endl;
}
Jet.h
#pragma once
#include "Plane.h"
//喷气式飞机
class Jet : public Plane {
virtual void fly(); //只有声明virtual函数才能产生多态,否则仍然调用父类方法
virtual void land();
};
Jet.cpp
#include "Jet.h"
#include <iostream>
using namespace std;
void Jet::fly() {
cout << "喷气式飞机在跑道上起飞..." << endl;
}
void Jet::land() {
cout << "喷气式飞机在跑道上降落..." << endl;
}
Copter.h
#pragma once
#include "Plane.h"
//普通飞机
class Copter : public Plane {
public:
virtual void fly();
virtual void land();
};
Copter.cpp
#include "Copter.h"
#include <iostream>
using namespace std;
void Copter::fly() {
cout << "直升飞机在原地起飞..." << endl;
}
void Copter::land() {
cout << "直升飞机降落在女神的屋顶..." << endl;
}
纯虚函数(抽象类)
//纯虚函数(抽象类)
//1.当一个类具有一个纯虚函数,这个类就是抽象类
//2.抽象类不能实例化对象
//3.子类继承抽象类,必须要实现纯虚函数,如果没有,子类也是抽象类
//抽象类的作用:为了继承约束,根本不知道未来的实现
//形状
class Shape {
public:
//纯虚函数
virtual void sayArea() = 0;
void print() {
cout << "hi" << endl;
}
};
//圆
class Circle : public Shape {
public:
Circle(int r) {
this->r = r;
}
void sayArea() {
cout << "圆的面积:" << (3.14 * r * r) << endl;
}
private:
int r;
};
void main() {
//Shape s;
Circle c(10);
system("pause");
}
//接口(只是逻辑上的划分,语法上跟抽象类的写法没有区别)
//可以当做一个接口
class Drawble {
virtual void draw();
};
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/android-ndk-basic-cpp-inheritance-polymorphic/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

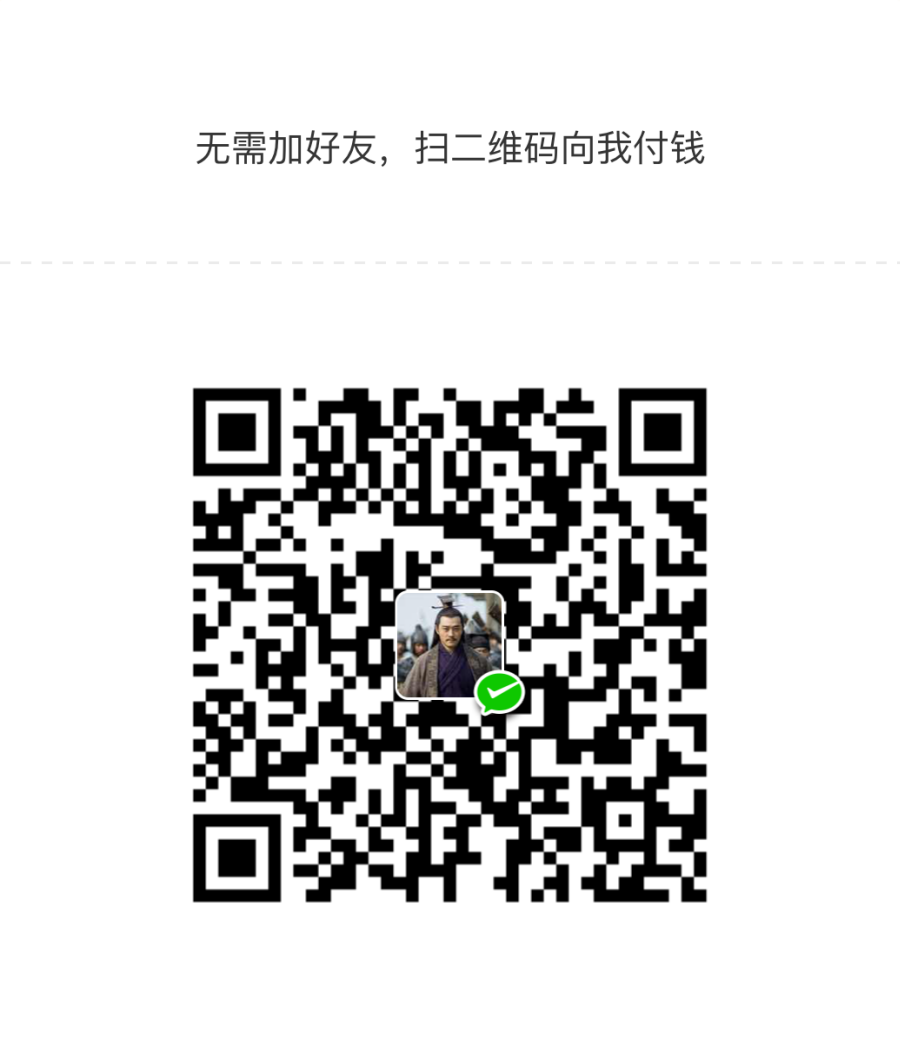
Android NDK基础21:C++_继承_多态
继承
#include <iostream>
//代码的重用性
using namespace std;
//人类
class Human {
public:
void say() {
cout << "说话&……

文章目录
关闭
共有 0 条评论