React Native学习之调用原生方法的步骤
React Native调用原生的方法,本文适配Android原生与RN的混合开发,步骤如下:
(1)使用 Android Studio 打开一个已存在的项目,选择RN项目中的android/build.gradle
文件
(2)在Android原生这边创建一个类继承ReactContextBaseJavaModule
,这个类里面存放需要被RN调用的方法,封装成一个原生模块
public class MyNativeModule extends ReactContextBaseJavaModule {
private ReactApplicationContext context;
public MyNativeModule(ReactApplicationContext reactContext) {
super(reactContext);
context = reactContext;
}
@Override
public String getName() {
//一定要返回一个名字,在RN代码里面需要使用这个名字来调用该类的方法
return "MyNativeModule";
}
//函数不能有返回值,因为被调用的原生代码是异步的,原生代码执行结束之后只能通过回调函数或者发送消息给RN
//必须声明ReactMethod注解
@ReactMethod
public void rnCallNative(String msg) {
Toast.makeText(context, msg, Toast.LENGTH_LONG).show();
}
}
(3)在Android原生这边创建一个类实现接口ReactPackage包管理器,并把第二步创建的类添加到原生模块(NativeModule)列表里
public class MyReactPackage implements ReactPackage {
@Override
public List<NativeModule> createNativeModules(ReactApplicationContext reactContext) {
List<NativeModule> modules = new ArrayList<>();
modules.add(new MyNativeModule(reactContext));
return modules;
}
@Override
public List<Class<? extends JavaScriptModule>> createJSModules() {
return Collections.emptyList();
}
@Override
public List<ViewManager> createViewManagers(ReactApplicationContext reactContext) {
return Collections.emptyList();
}
}
(4)将第三步创建的包管理器添加到ReactPackage列表里(MainApplication类的getPackages方法里)
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new MyReactPackage()
);
}
(5)编译原生应用
react-native run-android
报错:outDexFolder must be a folder
解决方法:重新编译
报错:Failed to create \AwesomeProject\android\app\build\intermediate s\debug\merging
解决方法:重新编译
(6)在RN中去调用原生模块
import {NativeModules} from 'react-native';
NativeModules.MyNativeModule.rnCallNative('React Native 调用原生模块的方法\nPowerd by RNAPP.CC');
源码:
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View,
NativeModules
} from 'react-native';
class RNAPP extends Component {
render() {
return (
<View style={styles.container}>
<Text style={styles.welcome}>
Welcome to React Native!
</Text>
<Text style={styles.welcome}>
React Native混合原生开发
</Text>
<Text style={styles.instructions}>
Powered by rnapp.cc
</Text>
<Text onPress={this.callNative.bind(this)} style={styles.btn}>调用原生方法</Text>
</View>
);
}
callNative() {
NativeModules.MyNativeModule.rnCallNative('React Native 调用原生模块的方法\nPowered by RNAPP.CC');
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
btn: {
marginTop: 50,
marginLeft: 10,
marginRight: 10,
width: 200,
height: 35,
backgroundColor: '#3BC1FF',
color: '#fff',
lineHeight: 24,
fontWeight: 'bold',
textAlign: 'center',
textAlignVertical:'center'
},
});
AppRegistry.registerComponent('RNAPP', () => RNAPP);
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/react-native-learning-call-native-methods-steps/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

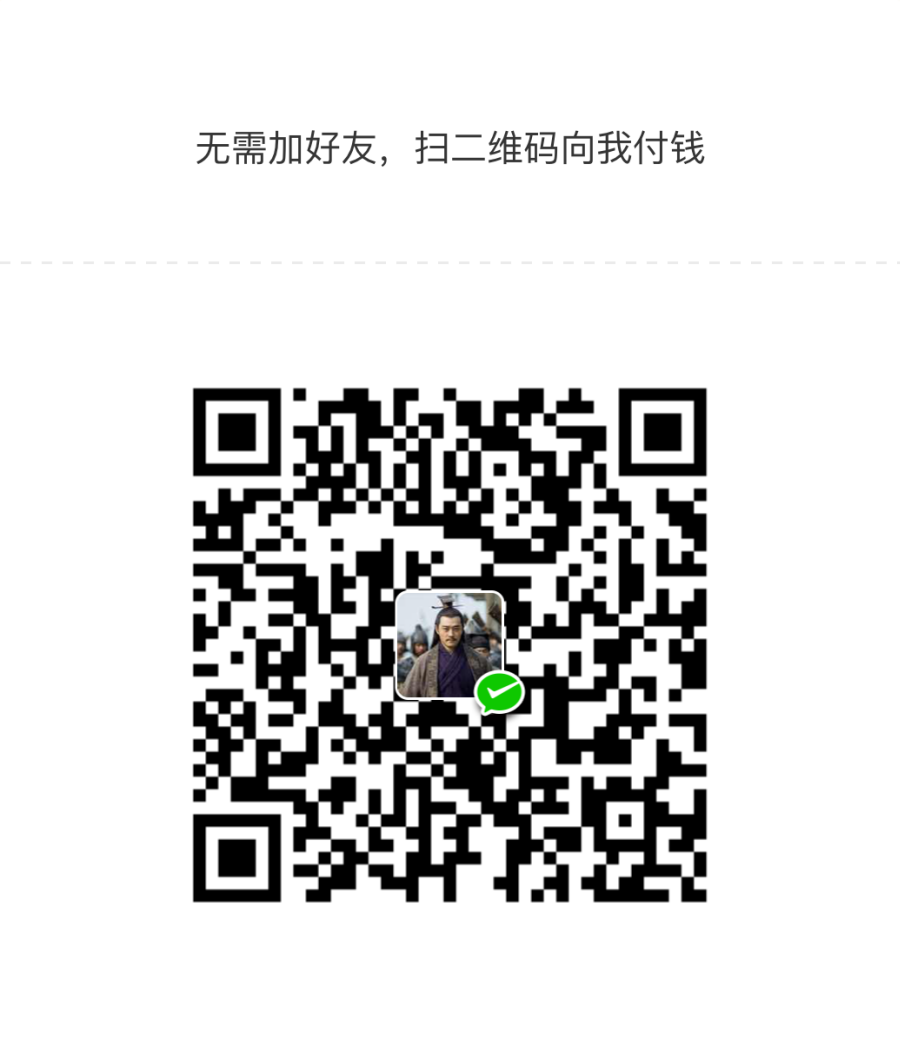

共有 0 条评论