React Native学习之TextInput组件
TextInput组件
TextInput:文本输入框,文本编辑框
TextInput的主要属性
- autoCapitalize:枚举类型,可选值有none sentences words characters 当用户输入时,用于提示
- placeholder:占位符,在输入前显示的文本内容
- value:文本输入框的默认值
- placeholderTextColor:占位符文本的颜色
- password:boolean类型,true表示密码输入显示*
- multiline:多行输入
- editable:文本框是否可输入
- autoFocus:自动聚焦
- clearButtonMode:枚举类型,never while-editing unless-editing always 用于显示清除按钮
- maxLength:能够输入的最长字符数
- enablesReturnKeyAutomatically:如果为true 表示没有文本时键盘是不能有返回键的,其默认值为false
- returnKeyType:枚举类型 default go google join next route search send yahoo done emergency-call 表示软键盘返回键显示的字符串
- secureTextEntry:隐藏输入内容,默认值为false
TextInput的主要事件
- onChangeText:当文本输入框的内容变化时,调用改函数;onChangeText接收一个文本的参数对象
- onChange:当文本变化时,调用该函数
- onEndEditing:当结束编辑时,调用改函数
- onBlur:失去焦点触发事件
- onFocus:获得焦点时触发事件
- onSubmitEditing:当结束编辑后,点击键盘的提交按钮触发该事件
案例:搜索框
/**
* Sample React Native App
* https://github.com/facebook/react-native
*/
'use strict';
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
TextInput,
View
} from 'react-native';
class RNAPP extends Component {
render() {
return (
<View style={[styles.flex,styles.topStatus]}>
<Search></Search>
</View>
);
}
}
class Search extends Component {
render(){
return(
<View style={[styles.flex,styles.flexDirection]}>
<View style={[styles.flex,styles.input]}>
<TextInput returnKeyType="search" />
</View>
<View style={styles.btn}>
<Text style={styles.search}>搜索</Text>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
flex:{
flex:1,
},
flexDirection:{
flexDirection:'row',
},
topStatus:{
marginTop:25,
},
input:{
height:45,
borderColor:'red',
borderWidth:1,
marginLeft:10,
paddingLeft:10,
borderRadius:5,
},
btn:{
width:45,
marginLeft:-5,
marginRight:5,
backgroundColor:'#23BEFF',
height:45,
justifyContent:'center',
alignItems:'center',
},
search:{
color:'#fff',
fontSize:15,
fontWeight:'bold',
},
});
AppRegistry.registerComponent('RNAPP', () => RNAPP);
TextInput自动提示的搜索框
搜索框加上自动提示的功能
很多app的搜索都是这样的:我们输入一个关键词的时候,会列出相关的搜索结果列表,一般情况下,完成该功能需要一个搜索服务,返回N条结果,并将其展示出来,这里我们用静态数据模拟出来。
/**
* Sample React Native App
* https://github.com/facebook/react-native
*/
'use strict';
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
PixelRatio,
ScrollView,
Text,
TextInput,
View
} from 'react-native';
var onePT = 1 / PixelRatio.get();
class RNAPP extends Component {
render() {
return (
<View style={[styles.flex, styles.topStatus]}>
<Search></Search>
</View>
);
}
}
class Search extends Component {
constructor(props) {
super(props);
this.state = {
show: false,
value: null,
};
}
hide(text) {
this.setState({
show: false,
value: text,
});
}
getValue(text) {
this.setState({
show: true,
value: text,
});
}
render() {
return(
<View style={styles.flex}>
<View style={styles.flexDirection}>
<View style={[styles.flex, styles.input]}>
<TextInput
keyboardType="web-search"
placeholder="请输入关键词"
value={this.state.value}
onChangeText={this.getValue.bind(this)}
/>
</View>
<View style={styles.btn}>
<Text
style={styles.search}
onPress={this.hide.bind(this, this.state.value)}>
搜索
</Text>
</View>
</View>
{this.state.show?
<View style={styles.result}>
<Text
onPress={this.hide.bind(this, this.state.value + ' React Native')}
style={styles.item}
numberOfLines={1}>
{this.state.value} React Native
</Text>
<Text
onPress={this.hide.bind(this, this.state.value + ' 中国')}
style={styles.item}
numberOfLines={1}>
{this.state.value} 中国
</Text>
<Text
onPress={this.hide.bind(this, 'APP开发 ' + this.state.value + ' 综合商店')}
style={styles.item}
numberOfLines={1}>
APP开发 {this.state.value} 综合商店
</Text>
<Text
onPress={this.hide.bind(this, this.state.value + ' RNAPP.CC')}
style={styles.item}
numberOfLines={1}>
{this.state.value} RNAPP.CC
</Text>
<Text
onPress={this.hide.bind(this, 'RN学习 ' + this.state.value )}
style={styles.item}
numberOfLines={1}>
RN学习 {this.state.value}
</Text>
</View>
: null
}
</View>
);
}
}
const styles = StyleSheet.create({
item:{
fontSize:16,
paddingTop:5,
paddingBottom:10,
},
result:{
marginTop:onePT,
marginLeft:18,
marginRight:5,
height:200,
},
flex:{
flex:1,
},
flexDirection:{
flexDirection:'row',
},
topStatus:{
marginTop:25,
},
input:{
height:50,
borderColor:'red',
borderWidth:1,
marginLeft:10,
paddingLeft:5,
borderRadius:5,
},
btn:{
width:45,
marginLeft:-5,
marginRight:5,
backgroundColor:'#23BEFF',
height:50,
justifyContent:'center',
alignItems:'center',
},
search:{
color:'#fff',
fontSize:15,
fontWeight:'bold',
},
});
AppRegistry.registerComponent('RNAPP', () => RNAPP);
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/react-native-learning-textinput-component/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

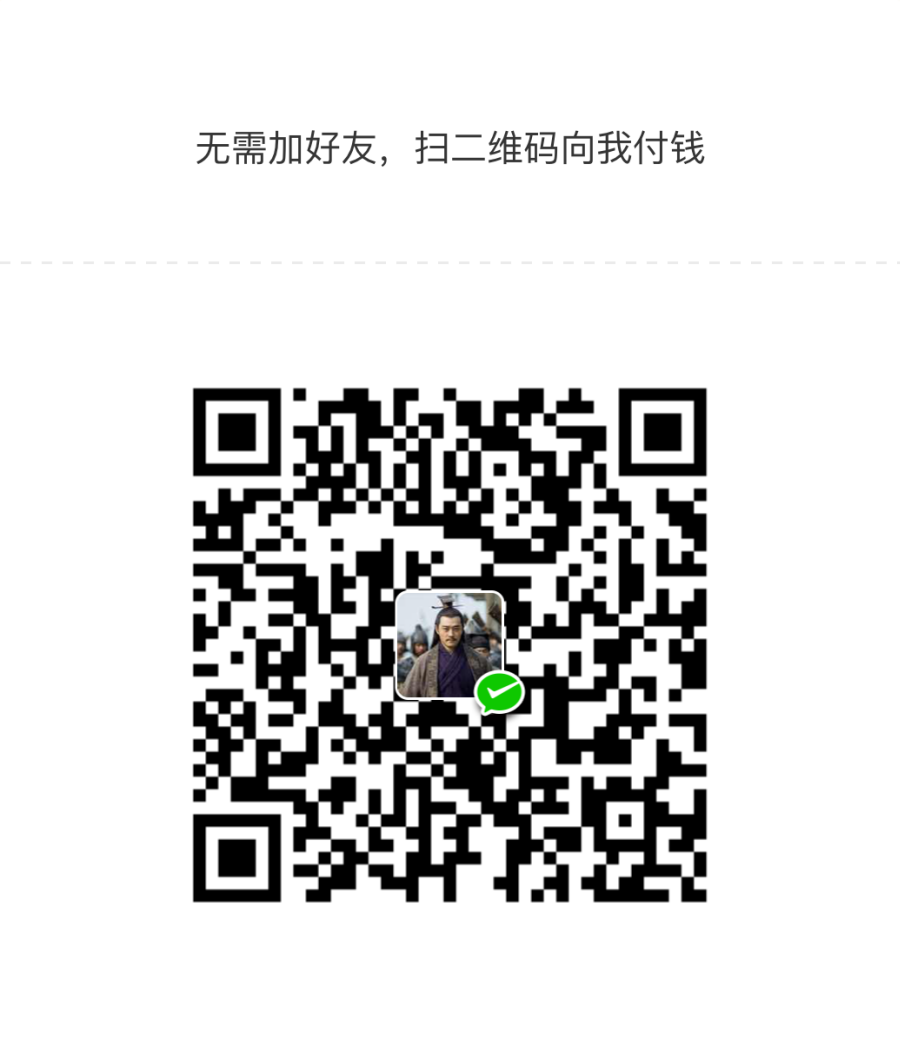
React Native学习之TextInput组件
TextInput组件
TextInput:文本输入框,文本编辑框
TextInput的主要属性
autoCapitalize:枚举类型,可选值有none sentences words characters 当用户输入时……

文章目录
关闭
共有 0 条评论