Swift UI – 使用表格组件(UITableView)实现单列表
注:代码已升级至Swift4
需求说明
(1)列表内容从 Controls.plist 文件中读取,类型为 Array
(2)点击列表项会弹出消息框显示该项信息
(3)按住列表项向左滑动,会出现删除按钮。点击删除即可删除该项
单元格复用机制
由于普通的表格视图中单元格形式一般都是相同的,所以本例采用了单元格复用机制,可以大大提高程序性能。
实现方式是初始化创建 UITableView
实例时使用 .register(UITableViewCell.self, forCellReuseIdentifier: "SwiftCell")
创建一个可供重用的 UITableViewCell
。并将其注册到UITableView
,ID为 SwiftCell
。下次碰到形式(或结构)相同的单元就可以直接使用UITableView
的dequeueReusableCell
方法从UITableView
中取出。
代码示例
ViewController.swift
import UIKit
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource {
var ctrlnames:[String]?
var tableView:UITableView?
override func loadView() {
super.loadView()
}
override func viewDidLoad() {
super.viewDidLoad()
//初始化数据,这一次数据,我们放在属性列表文件里
self.ctrlNames = NSArray(contentsOfFile:
Bundle.main.path(forResource: "Controls", ofType:"plist")!) as? Array
print(self.ctrlNames)
//创建表视图
self.tableView = UITableView(frame: self.view.frame, style:.plain)
self.tableView!.delegate = self
self.tableView!.dataSource = self
//创建一个重用的单元格
self.tableView!.register(UITableViewCell.self,
forCellReuseIdentifier: "SwiftCell")
self.view.addSubview(self.tableView!)
//创建表头标签
let frame = CGRect(x:0, y:0, width:self.view.bounds.size.width, height:30)
let headerLabel = UILabel(frame: frame)
headerLabel.backgroundColor = UIColor.black
headerLabel.textColor = UIColor.white
headerLabel.numberOfLines = 0
headerLabel.lineBreakMode = .byWordWrapping
headerLabel.text = "常见 UIKit 控件"
headerLabel.font = UIFont.italicSystemFont(ofSize: 20)
self.tableView!.tableHeaderView = headerLabel
}
//返回表格分区数,本例只有一个分区
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
//返回表格行数(也就是返回控件数)
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.ctrlNames!.count
}
//创建各单元显示内容(创建参数indexPath指定的单元)
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath)
-> UITableViewCell {
//为了提供表格显示性能,已创建完成的单元需重复使用
let identify:String = "SwiftCell"
//同一形式的单元格重复使用,在声明时已注册
let cell = tableView.dequeueReusableCell(withIdentifier: identify,
for: indexPath)
cell.accessoryType = .disclosureIndicator
cell.textLabel?.text = self.ctrlNames![indexPath.row]
return cell
}
// UITableViewDelegate 方法,处理列表项的选中事件
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
self.tableView!.deselectRow(at: indexPath, animated: true)
let itemString = self.ctrlNames![indexPath.row]
let alertController = UIAlertController(title: "提示!",
message: "你选中了【\(itemString)】", preferredStyle: .alert)
let okAction = UIAlertAction(title: "确定", style: .default, handler: nil)
alertController.addAction(okAction)
self.present(alertController, animated: true, completion: nil)
}
//滑动删除必须实现的方法
func tableView(_ tableView: UITableView,
commit editingStyle: UITableViewCellEditingStyle,
forRowAt indexPath: IndexPath) {
print("删除\(indexPath.row)")
let index = indexPath.row
self.ctrlNames?.remove(at: index)
self.tableView?.deleteRows(at: [indexPath], with: .top)
}
//滑动删除
func tableView(_ tableView: UITableView, editingStyleForRowAt indexPath: IndexPath)
-> UITableViewCellEditingStyle {
return UITableViewCellEditingStyle.delete
}
//修改删除按钮的文字
func tableView(_ tableView: UITableView, titleForDeleteConfirmationButtonForRowAt indexPath: IndexPath) -> String? {
return "删除"
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}
Controls.plist
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN"
"http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<array>
<string>标签 UILabel</string>
<string>文本框 UITextField</string>
<string>按钮 UIButton</string>
<string>开关按钮 UISwitch</string>
<string>分段控件 UISegmentControl</string>
<string>图像 UIImageView</string>
<string>进度条 UIProgressView</string>
<string>滑动条 UISlider</string>
<string>警告框 UIAlertView</string>
</array>
</plist>
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/swift-ui-use-table-component-uitableview-to-implement-single-list/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

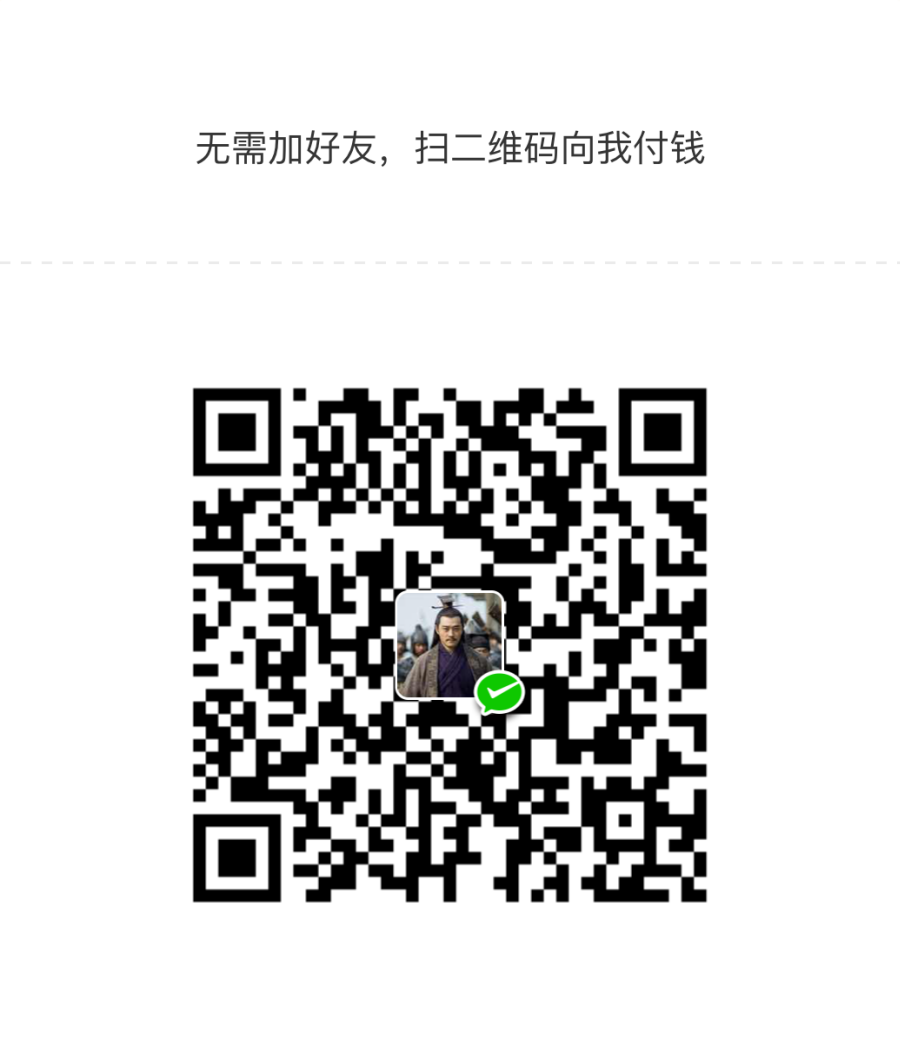

共有 0 条评论