Spring Boot内置定时任务
在我们开发项目过程中,经常需要定时任务来帮助我们来做一些内容,Spring Boot默认已经帮我们实现,只需要添加相应的注解即可
pom 包配置
pom 包里面只需要引入Spring Boot Starter
包即可
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
启动类启用定时
在Application启动类上面加上@EnableScheduling
即可开启定时
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
创建定时任务实现类
定时任务1:
@Component
public class SchedulerTask {
private int count = 0;
@Scheduled(cron="*/6 * * * * ?")
private void process(){
System.out.println("This is scheduler task runing: " + (count++));
}
}
定时任务2:
@Component
public class Scheduler2Task {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
@Scheduled(fixedRate = 6000)
public void reportCurrentTime() {
System.out.println("现在时间: " + dateFormat.format(new Date()));
}
}
结果如下:
现在时间: 10:29:32
This is scheduler task runing: 0
现在时间: 10:29:38
This is scheduler task runing: 1
现在时间: 10:29:44
This is scheduler task runing: 2
现在时间: 10:29:50
This is scheduler task runing: 3
现在时间: 10:29:56
This is scheduler task runing: 4
现在时间: 10:30:02
This is scheduler task runing: 5
现在时间: 10:30:08
参数说明
@Scheduled
参数可以接受两种定时的设置,一种是我们常用的crond语法cron="*/6 * * * * ?"
,一种是fixedRate = 6000
,两种都表示每隔六秒打印一下内容。
fixedRate
说明
@Scheduled(fixedRate = 6000)
:上一次开始执行时间点之后6秒再执行@Scheduled(fixedDelay = 6000)
:上一次执行完毕时间点之后6秒再执行@Scheduled(initialDelay=1000, fixedRate=6000)
:第一次延迟1秒后执行,之后按 fixedRate 的规则每6秒执行一次
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/09/spring-boot-built-in-scheduled-tasks/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

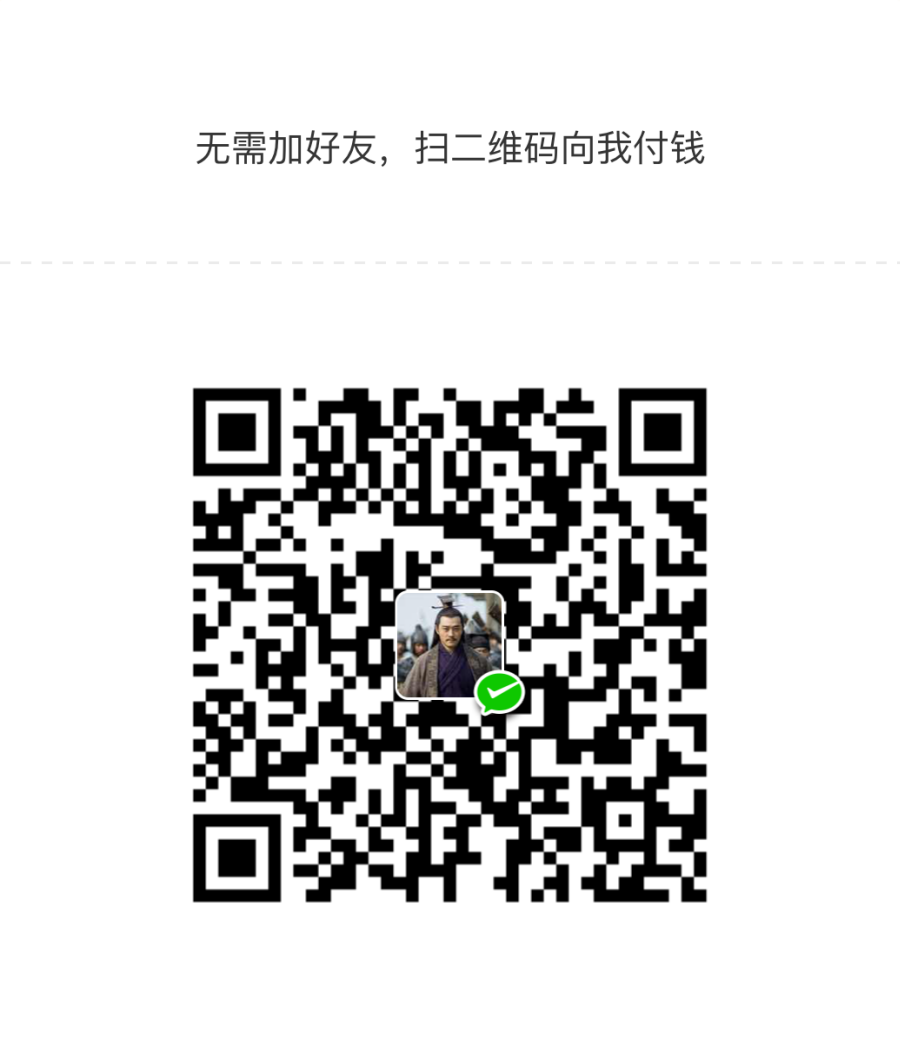
Spring Boot内置定时任务
在我们开发项目过程中,经常需要定时任务来帮助我们来做一些内容,Spring Boot默认已经帮我们实现,只需要添加相应的注解即可
pom 包配置
pom 包里面只需要引……

文章目录
关闭
共有 0 条评论