Spring Boot上传文件到腾讯云储存
腾讯云平台操作
登录到腾讯云控制台 -> 对象存储 -> 创建存储桶(访问权限设置为公有读私有写)
空间名称 appblog-1253563213
所属地域 广州(华南) (ap-guangzhou)
访问域名 https://appblog-1253563213.cos.ap-guangzhou.myqcloud.com
秘钥管理 -> 新建云API秘钥
SecretId: AKIDhBQQAjuGA6MehUITeZDbjcGessc9st88
SecretKey: L2JK6JHEySV3LgmZSWehXAYsxoQn75kA
Spring boot项目集成
Maven依赖
<!-- https://mvnrepository.com/artifact/com.qcloud/cos_api -->
<dependency>
<groupId>com.qcloud</groupId>
<artifactId>cos_api</artifactId>
<version>5.5.1</version>
</dependency>
配置信息
tencent:
#腾讯云存储所需信息
cloud:
#SecretId
accessKey: AKIDhBQQAjuGA6MehUITeZDbjcGessc9st88
#SecretKey
secretKey: L2JK6JHEySV3LgmZSWehXAYsxoQn75kA
#所属区域
bucket: ap-guangzhou
#存储桶名称
bucketName: appblog-1253563213
#访问域名
path: https://appblog-1253563213.cos.ap-guangzhou.myqcloud.com
#路径前缀
prefix: appblog
@Getter
@Setter
@Configuration
public class TencentConfig {
@Value("${tencent.cloud.accessKey}")
private String accessKey;
@Value("${tencent.cloud.secretKey}")
private String secretKey;
@Value("${tencent.cloud.bucket}")
private String bucket;
@Value("${tencent.cloud.bucketName}")
private String bucketName;
@Value("${tencent.cloud.path}")
private String path;
@Value("${tencent.cloud.prefix}")
private String prefix;
}
文件上传控制器
@Controller
@Slf4j
@RequestMapping(value = "/upload/tencent")
public class TencentUploadController {
@Autowired
private TencentConfig tencentConfig;
/**
* 上传道腾讯云服务器(https://cloud.tencent.com/document/product/436/10199)
* @return
*/
@RequestMapping(value = "/single", method = RequestMethod.POST)
@ResponseBody
public Object Upload(@RequestParam(value = "file") MultipartFile file, HttpSession session) {
if (file == null) {
return new UploadMsg(0, "文件为空", null);
}
String oldFileName = file.getOriginalFilename();
String eName = oldFileName.substring(oldFileName.lastIndexOf("."));
String newFileName = UUID.randomUUID() + eName;
Calendar cal = Calendar.getInstance();
int year = cal.get(Calendar.YEAR);
int month = cal.get(Calendar.MONTH) + 1;
int day = cal.get(Calendar.DATE);
// 1 初始化用户身份信息(secretId, secretKey)
COSCredentials cred = new BasicCOSCredentials(tencentConfig.getAccessKey(), tencentConfig.getSecretKey());
// 2 设置bucket的区域, COS地域的简称请参照 https://cloud.tencent.com/document/product/436/6224
ClientConfig clientConfig = new ClientConfig(new Region(tencentConfig.getBucket()));
// 3 生成cos客户端
COSClient cosclient = new COSClient(cred, clientConfig);
// bucket的命名规则为{name}-{appid} ,此处填写的存储桶名称必须为此格式
String bucketName = tencentConfig.getBucketName();
// 简单文件上传, 最大支持 5 GB, 适用于小文件上传, 建议 20 M 以下的文件使用该接口
// 大文件上传请参照 API 文档高级 API 上传
File localFile = null;
try {
localFile = File.createTempFile("temp", null);
file.transferTo(localFile);
// 指定要上传到 COS 上的路径
String key = "/" + tencentConfig.getPrefix() + "/" + year + "/" + month + "/" + day + "/" + newFileName;
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, key, localFile);
PutObjectResult putObjectResult = cosclient.putObject(putObjectRequest);
log.info(putObjectResult.getContentMd5());
log.info(putObjectResult.getDateStr());
log.info(putObjectResult.getETag());
log.info(putObjectResult.getRequestId());
//{"status":1,"msg":"上传成功","path":"https://appblog-1253563213.cos.ap-guangzhou.myqcloud.com/appblog/2019/2/20/3f934239-2c92-4da1-bb80-896299fcdcdf.png"}
return new UploadMsg(1, "上传成功", tencentConfig.getPath() + putObjectRequest.getKey());
} catch (IOException e) {
return new UploadMsg(-1, e.getMessage(), null);
} finally {
// 关闭客户端(关闭后台线程)
cosclient.shutdown();
}
}
}
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
public class UploadMsg {
public int status;
public String msg;
public String path;
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/09/spring-boot-upload-files-to-tencent-cloud-storage/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

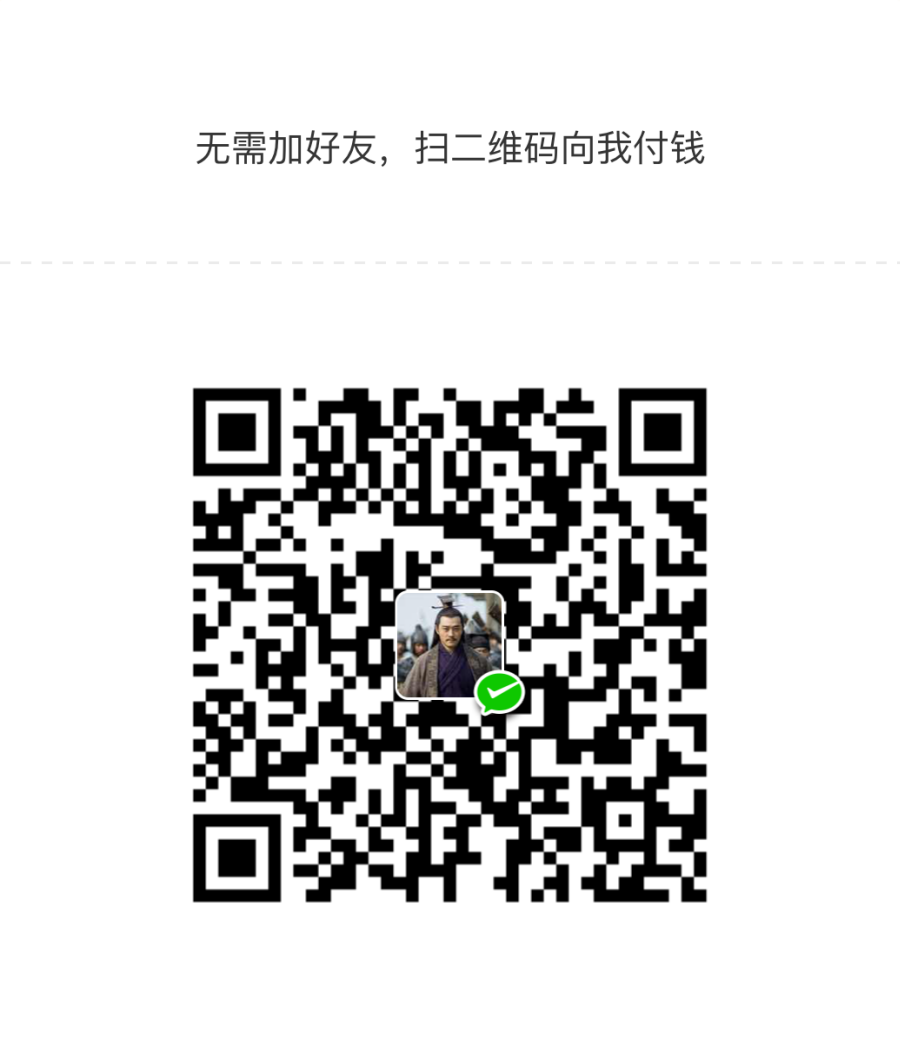
Spring Boot上传文件到腾讯云储存
腾讯云平台操作
登录到腾讯云控制台 -> 对象存储 -> 创建存储桶(访问权限设置为公有读私有写)
空间名称 appblog-1253563213
所属地域 广州(华……

文章目录
关闭
共有 0 条评论