GreenDao 3 的配置及使用
GreenDao是一个优质的开源数据库,每秒支持上千次的增删改查,效率非常高。更多详细介绍:https://github.com/greenrobot/greenDAO
依赖配置
// In your root build.gradle file:
buildscript {
repositories {
jcenter()
mavenCentral() // add repository
}
dependencies {
classpath 'com.android.tools.build:gradle:3.1.1'
classpath 'org.greenrobot:greendao-gradle-plugin:3.2.2' // add plugin
}
}
// In your app projects build.gradle file:
apply plugin: 'com.android.application'
apply plugin: 'org.greenrobot.greendao' // apply plugin
android {
...
// 配置数据库
greendao {
// 数据库版本号
schemaVersion 1
// 数据库所在包
daoPackage 'me.yezhou.lib.app_base.db.dao'
// 生成的数据库文件的目录
targetGenDir 'src/main/java'
// 自动生成单元测试
// generateTests true
// 生成的单元测试目录
// targetGenDirTests
}
}
dependencies {
implementation 'org.greenrobot:greendao:3.2.2' // add library
}
实体模型
全部配置成功之后,建立数据库实体模型
@Entity
public class Student {
@Id(autoincrement = true)
private Long _id; //务必使用对象类型Long,不用基本数据类型long
@Unique
@NotNull
@Property(nameInDb = "username")
private String name;
@NotNull
private String age;
@Transient
private String info;
@Property(nameInDb = "country_id")
private Long countryId;
@ToOne(joinProperty = "countryId")
private Region country;
}
注解解析
@Entity
:标记该类为数据库实体模型,指示GreendDao生成代码@Id(autoincrement = true)
:配置id自增@Unique
:字段约束,保证值唯一@NotNull
:字段不允许为空@Property(nameInDb = "username")
:设置该列名非默认,采用自定义@Transient
:设置该键在数据库中排除@ToOne(joinProperty = "countryId")
:关联另一实体并指定外联键
构建代码
完成创建之后,点击Sync Progect
或GreenDao自带的Gradle命令,GreenDao将会自动生成代码:
DaoMaster
:该类保存了数据库对象(SQLiteDatabase),它的内部类OpenHelper和DevOpenHelper是SQLiteOpenHelper的实现DaoSession
:提供对数据库进行增删改查的方法StudentDao
:GreenDao对应于每个实体生成的类,即配置@Entity
标注的类
特别注意,不要轻易改动自动生成的代码。
初始化
初始化核心对象
public class GreenDaoHelper {
private static DaoMaster.DevOpenHelper mHelper;
private static SQLiteDatabase mDatabase;
private static DaoMaster mDaoMaster;
private static DaoSession mDaoSession;
/**
* 设置GreenDao
*/
public static void initDatabase() {
mHelper = new DaoMaster.DevOpenHelper(MyApp.mContext, "student.db", null);
mDatabase = mHelper.getWritableDatabase();
mDaoMaster = new DaoMaster(mDatabase);
mDaoSession = mDaoMaster.newSession();
}
public static DaoSession getDaoSession() {
return mDaoSession;
}
public static SQLiteDatabase getDatabase() {
return mDatabase;
}
}
在Appliction中应用
public class MyApp extends Application {
public static Context mContext;
@Override
public void onCreate() {
super.onCreate();
mContext = getApplicationContext();
GreenDaoHelper.initDatabase();
}
}
数据库操作
//获取数据库对象
mStudentDao = GreenDaoHelper.getDaoSession().getStudentDao();
//插入一条数据,传入null,id会自增
mStudentDao.insert(new Student(null, "Joe.Ye", "28"));
//删除一条数据
mStudentDao.delete(new Student(null, "Joe.Ye", "28"));
//根据主键删除一条数据
mStudentDao.deleteByKey(1L);
//删除全部数据
mStudentDao.deleteAll();
//修改一条数据
mStudentDao.update(new Student(null, "Joe.Ye", "25"));
//查询全部数据
mStudentDao.loadAll();
//查询一条数据,where中填写条件
mStudentDao.queryBuilder().where(StudentDao.Properties.Name.eq("Joe.Ye")).unique();
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/configuration-and-use-of-greendao-3/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

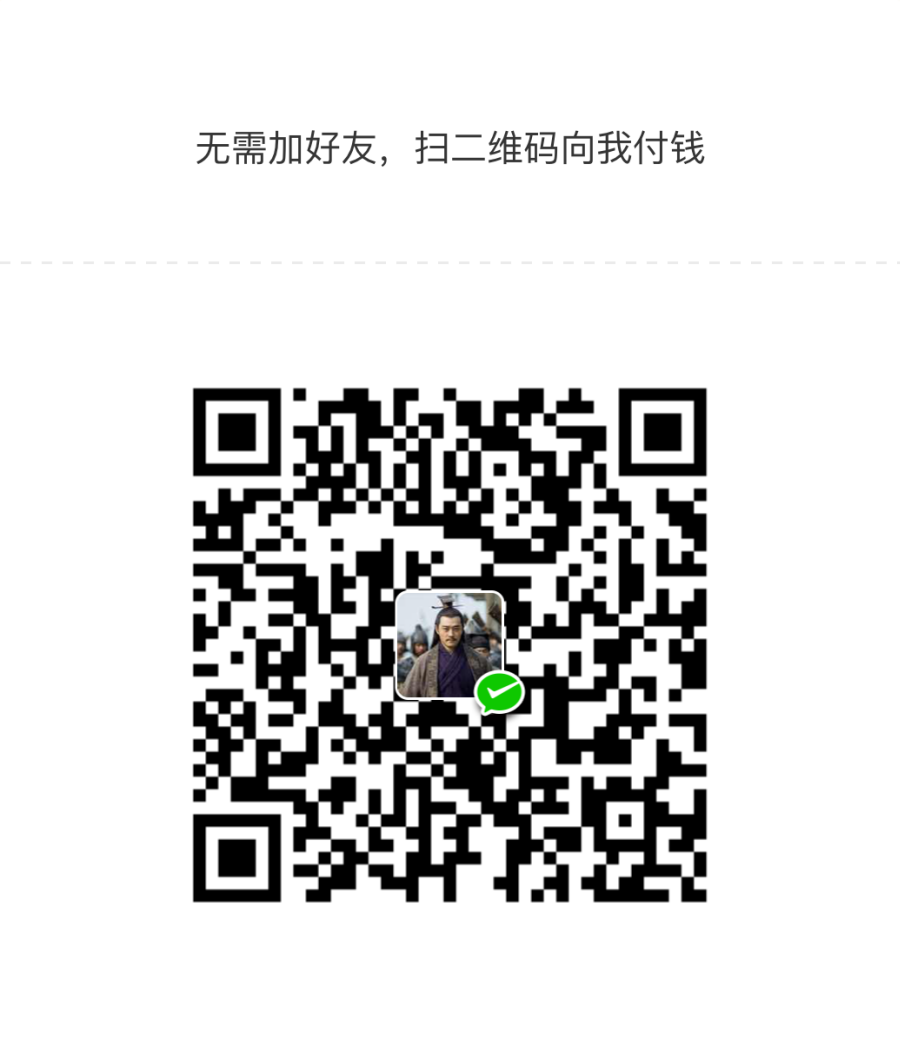
GreenDao 3 的配置及使用
GreenDao是一个优质的开源数据库,每秒支持上千次的增删改查,效率非常高。更多详细介绍:https://github.com/greenrobot/greenDAO
依赖配置
// In your root……

文章目录
关闭
共有 0 条评论