MyBatis mybatis-generator自定义plugin
字段添加注释
generatorConfig.xml添加自定义plugin示例
<!-- 生成的代码去掉注释 -->
<commentGenerator type="com.lianpay.globalpay.remit.common.plugin.CommentGenerator">
<property name="suppressAllComments" value="true" />
<property name="suppressDate" value="true"/>
</commentGenerator>
/**
* 生成model中,字段添加注释
*/
public class CommentGenerator extends DefaultCommentGenerator {
@Override
public void addFieldComment(Field field, IntrospectedTable introspectedTable, IntrospectedColumn introspectedColumn) {
super.addFieldComment(field, introspectedTable, introspectedColumn);
if (introspectedColumn.getRemarks() != null && !introspectedColumn.getRemarks().equals("")) {
field.addJavaDocLine("/**");
field.addJavaDocLine(" * " + introspectedColumn.getRemarks());
addJavadocTag(field, false);
field.addJavaDocLine(" */");
}
}
}
加密配置文件
/**
* 支持加密配置文件插件
*/
public class EncryptPropertyPlaceholderConfigurer extends PropertyPlaceholderConfigurer {
private Logger logger = LoggerFactory.getLogger(this.getClass());
private String[] propertyNames = {
"master.password", "slave.password", "generator.jdbc.password", "master.redis.password"
};
/**
* 解密指定propertyName的加密属性值
* @param propertyName
* @param propertyValue
* @return
*/
@Override
protected String convertProperty(String propertyName, String propertyValue) {
logger.info("Decrypt " + propertyName + "=" + propertyValue + " error!");
for (String p : propertyNames) {
if (p.equalsIgnoreCase(propertyName)) {
return AESUtil.AESDecode(propertyValue);
}
}
return super.convertProperty(propertyName, propertyValue);
}
}
Example类和model类实现序列化插件
/**
* Example类和model类实现序列化插件
*/
public class SerializablePlugin extends PluginAdapter {
private FullyQualifiedJavaType serializable = new FullyQualifiedJavaType("java.io.Serializable");
private FullyQualifiedJavaType gwtSerializable = new FullyQualifiedJavaType("com.google.gwt.user.client.rpc.IsSerializable");
private boolean addGWTInterface;
private boolean suppressJavaInterface;
public SerializablePlugin() {
}
public boolean validate(List<String> warnings) {
return true;
}
public void setProperties(Properties properties) {
super.setProperties(properties);
this.addGWTInterface = Boolean.valueOf(properties.getProperty("addGWTInterface")).booleanValue();
this.suppressJavaInterface = Boolean.valueOf(properties.getProperty("suppressJavaInterface")).booleanValue();
}
public boolean modelBaseRecordClassGenerated(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
this.makeSerializable(topLevelClass, introspectedTable);
return true;
}
public boolean modelPrimaryKeyClassGenerated(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
this.makeSerializable(topLevelClass, introspectedTable);
return true;
}
public boolean modelRecordWithBLOBsClassGenerated(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
this.makeSerializable(topLevelClass, introspectedTable);
return true;
}
protected void makeSerializable(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
if(this.addGWTInterface) {
topLevelClass.addImportedType(this.gwtSerializable);
topLevelClass.addSuperInterface(this.gwtSerializable);
}
if(!this.suppressJavaInterface) {
topLevelClass.addImportedType(this.serializable);
topLevelClass.addSuperInterface(this.serializable);
Field field = new Field();
field.setFinal(true);
field.setInitializationString("1L");
field.setName("serialVersionUID");
field.setStatic(true);
field.setType(new FullyQualifiedJavaType("long"));
field.setVisibility(JavaVisibility.PRIVATE);
this.context.getCommentGenerator().addFieldComment(field, introspectedTable);
topLevelClass.addField(field);
}
}
/**
* 添加给Example类序列化的方法
* @param topLevelClass
* @param introspectedTable
* @return
*/
@Override
public boolean modelExampleClassGenerated(TopLevelClass topLevelClass,IntrospectedTable introspectedTable){
makeSerializable(topLevelClass, introspectedTable);
for (InnerClass innerClass : topLevelClass.getInnerClasses()) {
if ("GeneratedCriteria".equals(innerClass.getType().getShortName())) {
innerClass.addSuperInterface(serializable);
}
if ("Criteria".equals(innerClass.getType().getShortName())) {
innerClass.addSuperInterface(serializable);
}
if ("Criterion".equals(innerClass.getType().getShortName())) {
innerClass.addSuperInterface(serializable);
}
}
return true;
}
}
MySQL分页插件
/**
* MySQL分页插件
*/
public class PaginationPlugin extends PluginAdapter {
@Override
public boolean validate(List<String> list) {
return true;
}
/**
* 为每个Example类添加limit和offset属性和set、get方法
*/
@Override
public boolean modelExampleClassGenerated(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
PrimitiveTypeWrapper integerWrapper = FullyQualifiedJavaType.getIntInstance().getPrimitiveTypeWrapper();
Field limit = new Field();
limit.setName("limit");
limit.setVisibility(JavaVisibility.PRIVATE);
limit.setType(integerWrapper);
topLevelClass.addField(limit);
Method setLimit = new Method();
setLimit.setVisibility(JavaVisibility.PUBLIC);
setLimit.setName("setLimit");
setLimit.addParameter(new Parameter(integerWrapper, "limit"));
setLimit.addBodyLine("this.limit = limit;");
topLevelClass.addMethod(setLimit);
Method getLimit = new Method();
getLimit.setVisibility(JavaVisibility.PUBLIC);
getLimit.setReturnType(integerWrapper);
getLimit.setName("getLimit");
getLimit.addBodyLine("return limit;");
topLevelClass.addMethod(getLimit);
Field offset = new Field();
offset.setName("offset");
offset.setVisibility(JavaVisibility.PRIVATE);
offset.setType(integerWrapper);
topLevelClass.addField(offset);
Method setOffset = new Method();
setOffset.setVisibility(JavaVisibility.PUBLIC);
setOffset.setName("setOffset");
setOffset.addParameter(new Parameter(integerWrapper, "offset"));
setOffset.addBodyLine("this.offset = offset;");
topLevelClass.addMethod(setOffset);
Method getOffset = new Method();
getOffset.setVisibility(JavaVisibility.PUBLIC);
getOffset.setReturnType(integerWrapper);
getOffset.setName("getOffset");
getOffset.addBodyLine("return offset;");
topLevelClass.addMethod(getOffset);
return true;
}
/**
* 为Mapper.xml的selectByExample添加limit,offset
*/
@Override
public boolean sqlMapSelectByExampleWithoutBLOBsElementGenerated(XmlElement element,
IntrospectedTable introspectedTable) {
XmlElement ifLimitNotNullElement = new XmlElement("if");
ifLimitNotNullElement.addAttribute(new Attribute("test", "limit != null"));
XmlElement ifOffsetNotNullElement = new XmlElement("if");
ifOffsetNotNullElement.addAttribute(new Attribute("test", "offset != null"));
ifOffsetNotNullElement.addElement(new TextElement("limit ${offset}, ${limit}"));
ifLimitNotNullElement.addElement(ifOffsetNotNullElement);
XmlElement ifOffsetNullElement = new XmlElement("if");
ifOffsetNullElement.addAttribute(new Attribute("test", "offset == null"));
ifOffsetNullElement.addElement(new TextElement("limit ${limit}"));
ifLimitNotNullElement.addElement(ifOffsetNullElement);
element.addElement(ifLimitNotNullElement);
return true;
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/mybatis-mybatis-generator-custom-plugin/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

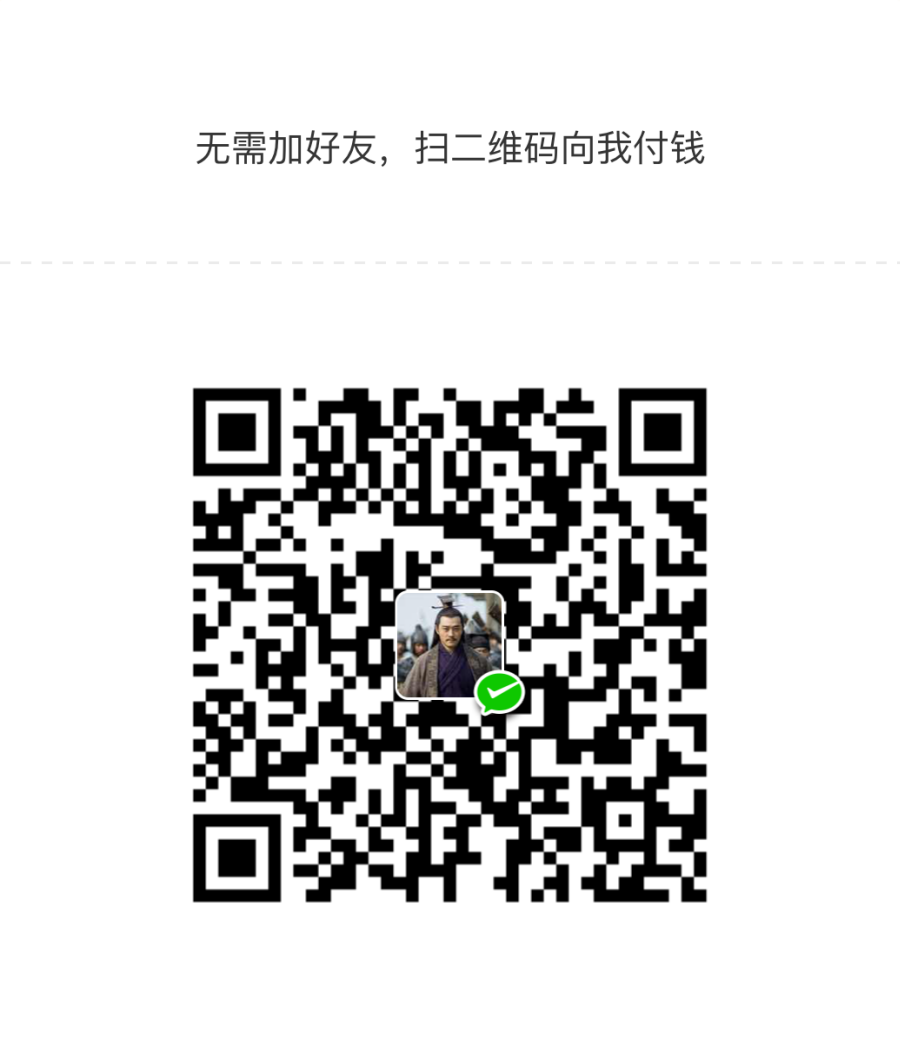
MyBatis mybatis-generator自定义plugin
字段添加注释
generatorConfig.xml添加自定义plugin示例
<!-- 生成的代码去掉注释 -->
<commentGenerator type="com.lianpay.globalpay.remit.……

文章目录
关闭
共有 0 条评论