Spring Boot时间格式的时区转换
前言:需要做时区转换,知道北京为UTC+8,美西为UTC-8,世界标准时间为UTC,所以只需要知道时区是+8还是-8还是0即可,不需要使用"CTT"、"Asia/Shanghai"这种形式。
时区转换辅助类
@Component
public class DateHelper {
private SimpleDateFormat dateSdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private SimpleDateFormat dateUtcSdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private SimpleDateFormat dateE8Sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //北京时间
private SimpleDateFormat dateW8Sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //美国西部时间
@PostConstruct
public void init() {
dateUtcSdf.setTimeZone(TimeZone.getTimeZone("GMT+0"));
dateE8Sdf.setTimeZone(TimeZone.getTimeZone("GMT+8"));
dateW8Sdf.setTimeZone(TimeZone.getTimeZone("GMT-8"));
}
public String formatDate(Date date) {
if (date == null) return "";
return dateSdf.format(date);
}
public Date parseDate(String dateStr) throws ParseException {
if (StringUtils.isBlank(dateStr)) return null;
return dateSdf.parse(dateStr);
}
public Date parseUtcDate(String dateStr) throws ParseException {
if (StringUtils.isBlank(dateStr)) return null;
return dateUtcSdf.parse(dateStr);
}
public String formatUtcDate(Date date) {
if (date == null) return "";
return dateUtcSdf.format(date);
}
public Date parseE8Date(String dateStr) throws ParseException {
if (StringUtils.isBlank(dateStr)) return null;
return dateE8Sdf.parse(dateStr);
}
public String formatE8Date(Date date) {
if (date == null) return "";
return dateE8Sdf.format(date);
}
public Date parseW8Date(String dateStr) throws ParseException {
if (StringUtils.isBlank(dateStr)) return null;
return dateW8Sdf.parse(dateStr);
}
public String formatW8Date(Date date) {
if (date == null) return "";
return dateW8Sdf.format(date);
}
}
访问控制器
@RestController
@RequestMapping("datetime")
public class DateController {
@Autowired
private DateHelper dateHelper;
//北京时间
@GetMapping("tze8")
@ResponseBody
public String tze8(String datetime) {
try {
return dateHelper.formatE8Date(dateHelper.parseDate(datetime));
} catch (Exception e) {
return "";
}
}
//0时区
@GetMapping("tz0")
@ResponseBody
public String tz0(String datetime) {
try {
return dateHelper.formatUtcDate(dateHelper.parseDate(datetime));
} catch (Exception e) {
return "";
}
}
//美国西部时间
@GetMapping("tzw8")
@ResponseBody
public String tzw8(String datetime) {
try {
return dateHelper.formatW8Date(dateHelper.parseDate(datetime));
} catch (Exception e) {
return "";
}
}
}
访问测试
请求:http://127.0.0.1:8080/datetime/tz0?datetime=2019-05-20%2015:00:00
返回:2019-05-20 07:00:00
请求:http://127.0.0.1:8080/datetime/tze8?datetime=2019-05-20%2015:00:00
返回:2019-05-20 15:00:00
请求:http://127.0.0.1:8080/datetime/tzw8?datetime=2019-05-20%2015:00:00
返回:2019-05-19 23:00:00
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/spring-boot-time-format-time-zone-conversion/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

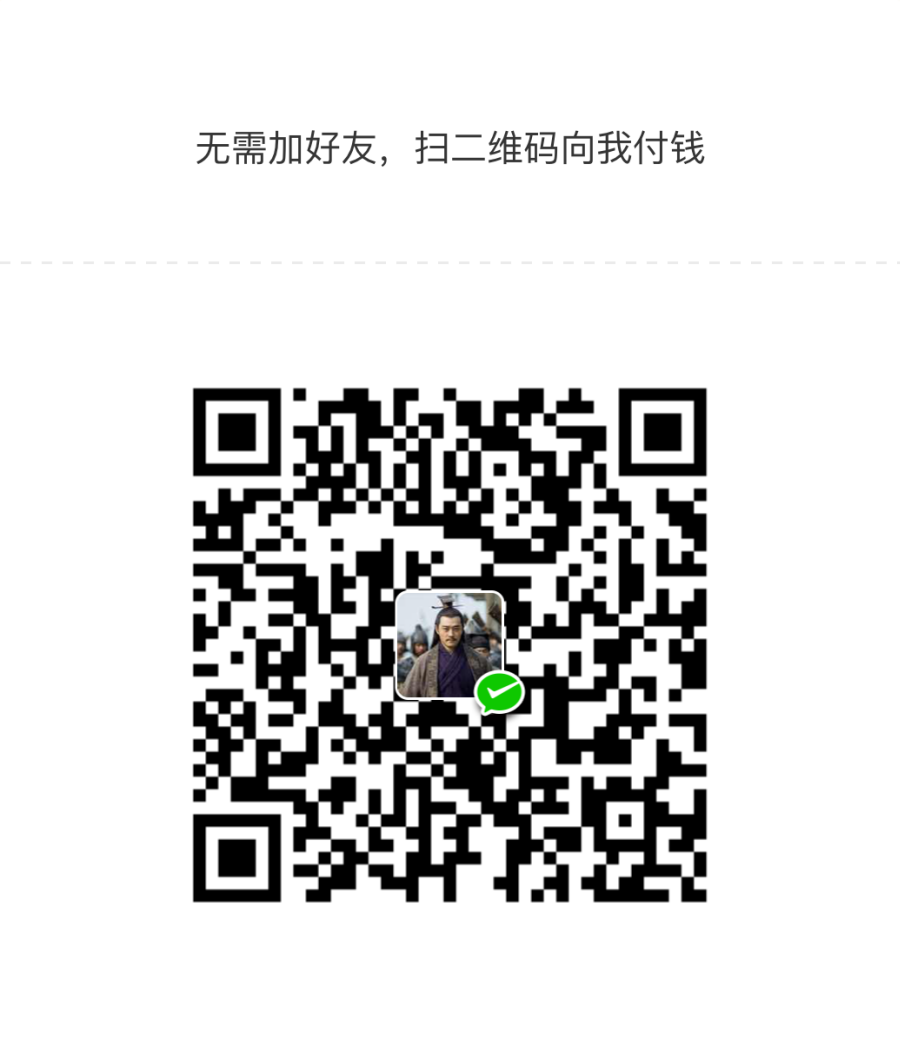

共有 0 条评论