Spring Boot使用RestTemplate批量下载文件
Spring Boot使用RestTemplate批量下载文件
private TestRestTemplate template = null;
@Before
public void testBefore() {
template = new TestRestTemplate();
}
@Test
public void getGoodsPicUrlList() throws IOException {
File dir = new File("E:/appblog.cn/goods/pic");
if (!dir.exists()) {
dir.mkdirs();
}
LitemallGoodsExample example = new LitemallGoodsExample();
List<LitemallGoods> goodsList = goodsMapper.selectByExampleSelective(example, LitemallGoods.Column.picUrl);
LitemallGoods goods;
for (int i = 0; i < goodsList.size(); i++) {
goods = goodsList.get(i);
String picUrl = goods.getPicUrl();
log.info(goods.getPicUrl());
HttpHeaders headers = new HttpHeaders();
HttpEntity<Resource> httpEntity = new HttpEntity<>(headers);
ResponseEntity<byte[]> response = template.exchange(picUrl, HttpMethod.GET, httpEntity, byte[].class);
log.info("状态码 >> {}", response.getStatusCodeValue());
//log.info(">> {}", response.getHeaders().getContentType());
//log.info(">> {}", response.getHeaders().getContentType().getSubtype());
try {
File file = new File(dir, picUrl.substring(picUrl.lastIndexOf('/')));
log.info(file.getAbsolutePath());
FileOutputStream fos = new FileOutputStream(file);
fos.write(response.getBody());
fos.flush();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void getGoodsGalleryList() throws IOException, InterruptedException {
File dir = new File("E:/litemall/goods/gallery");
if (!dir.exists()) {
dir.mkdirs();
}
LitemallGoodsExample example = new LitemallGoodsExample();
List<LitemallGoods> goodsList = goodsMapper.selectByExampleSelective(example, LitemallGoods.Column.gallery);
LitemallGoods goods;
for (int i = 0; i < goodsList.size(); i++) {
goods = goodsList.get(i);
String[] galleries = goods.getGallery();
for (int j = 0; j < galleries.length; j++) {
String gallery = galleries[j];
log.info(gallery);
HttpHeaders headers = new HttpHeaders();
HttpEntity<Resource> httpEntity = new HttpEntity<>(headers);
ResponseEntity<byte[]> response = template.exchange(gallery, HttpMethod.GET, httpEntity, byte[].class);
log.info("状态码 >> {}", response.getStatusCodeValue());
//log.info(">> {}", response.getHeaders().getContentType());
//log.info(">> {}", response.getHeaders().getContentType().getSubtype());
try {
File file = new File(dir, gallery.substring(gallery.lastIndexOf('/')));
log.info(file.getAbsolutePath());
FileOutputStream fos = new FileOutputStream(file);
fos.write(response.getBody());
fos.flush();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
Thread.sleep(100);
}
}
}
@Test
public void updateGoodsPicUrlList() throws IOException {
LitemallGoodsExample example = new LitemallGoodsExample();
List<LitemallGoods> goodsList = goodsMapper.selectByExampleSelective(example, LitemallGoods.Column.id, LitemallGoods.Column.picUrl);
LitemallGoods goods;
for (int i = 0; i < goodsList.size(); i++) {
goods = goodsList.get(i);
goods.setPicUrl(goods.getPicUrl().replace("http://www.yezhou.cc", "http://www.appblog.cn"));
goodsMapper.updateByPrimaryKeySelective(goods);
}
}
@Test
public void updateGoodsGalleryList() throws IOException, InterruptedException {
LitemallGoodsExample example = new LitemallGoodsExample();
List<LitemallGoods> goodsList = goodsMapper.selectByExampleSelective(example, LitemallGoods.Column.id, LitemallGoods.Column.gallery);
LitemallGoods goods;
for (int i = 0; i < goodsList.size(); i++) {
goods = goodsList.get(i);
String[] galleries = goods.getGallery();
String[] newGalleries = new String[galleries.length];
for (int j = 0; j < galleries.length; j++) {
newGalleries[j] = galleries[j].replace("http://www.yezhou.cc", "http://www.appblog.cn");
}
goods.setGallery(newGalleries);
goodsMapper.updateByPrimaryKeySelective(goods);
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/spring-boot-use-resttemplate-to-batch-download-files/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

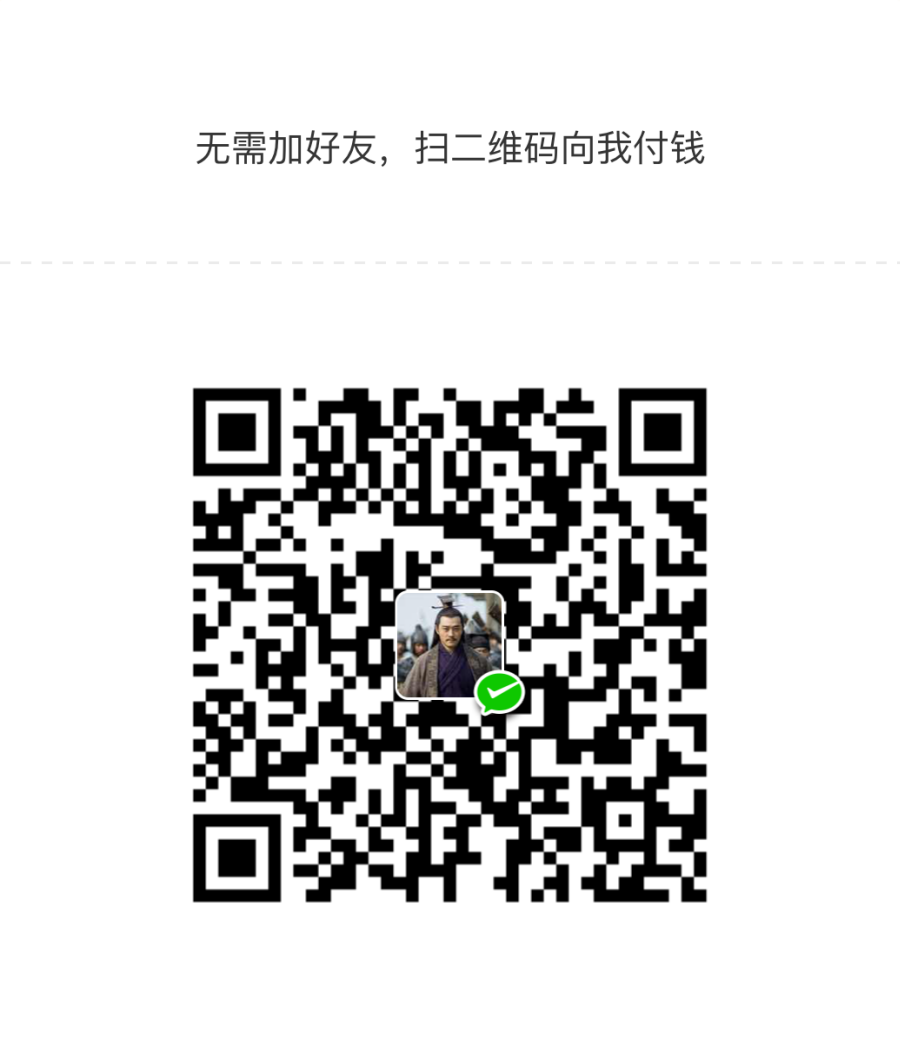
Spring Boot使用RestTemplate批量下载文件
Spring Boot使用RestTemplate批量下载文件
private TestRestTemplate template = null;
@Before
public void testBefore() {
template = new TestRestTe……

文章目录
关闭
共有 0 条评论