Webpack核心概念及入门
核心概念
Entry
| 代码的入口,打包的入口,单个或多个入口
Output
| 打包成的文件(bundle) 一个或多个 自定义规则
Loaders
| 处理文件,转化为模块
Plugins
| 可以参与整个打包的过程
| 打包优化和压缩
| 配置编译时的变量
Mode
Entry,Output
定义多个入口文件,输入多个文件
webpack.config.js
const webpack = require('webpack');
const {
resolve
} = require('path');
const config = {
entry: {
index:resolve(__dirname, 'src/index.js'),
vendor:resolve(__dirname, 'src/vendor.js')
},
output: {
path: resolve(__dirname, 'dist'),
filename: "[hash]-[name]-bundle.js"
},
mode: 'development'
}
module.exports = config
package.json
"scripts": {
"build":"webpack --config webpack.config.js"
}
Loader,Plugins
loader 让 webpack 能够去处理那些非 JavaScript 文件(webpack 自身只理解 JavaScript)。loader 可以将所有类型的文件转换为 webpack 能够处理的有效模块,然后你就可以利用 webpack 的打包能力,对它们进行处理。
例:加载css
npm i style-loader css-loader -S
const webpack = require('webpack');
const {resolve} = require('path');
const {CleanWebpackPlugin} = require('clean-webpack-plugin');
const config = {
entry: {
index:resolve(__dirname,'src/index.js'),
vendor:resolve(__dirname,'src/vendor.js')
},
output: {
path: resolve(__dirname, 'dist'),
filename: "[hash]-[name]-bundle.js"
},
plugins: [
new CleanWebpackPlugin()
],
+ module: {
+ rules: [
+ {
+ test: /\.css$/,
+ use: [
+ 'style-loader',
+ 'css-loader'
+ ]
+ }
+ ]
+ },
mode: 'development'
}
module.exports = config
//常用的loader
- 编译相关
babel-loader ts-loader
- 样式相关
style-loader css-loader less-loader postcss-loader sass-loader
- 文件相关
file-loader url-loader
代码压缩
module.exports = {
//...
optimization: {
minimize: true
}
};
入门示例
初始化webpack
npm init -y
npm i webpack webpack-cli -S
(1)新建webpack.config.js
const path = require('path');
const config = {
entry:path.join(__dirname,'src/index.js'),
output:{
/* 打包的文件名 */
filename:"bundle.js",
/* 打包文件的输出目录 */
path:path.join(__dirname,'dist')
},
mode:"development"
}
module.exports = config;
(2)修改package.json
文件
{
"name": "webpack-test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"build": "webpack --config webpack.config.js"
},
"dependencies": {
"webpack": "^4.41.0",
"webpack-cli": "^3.3.9",
}
}
(3)初次打包
npm run build
配置plugins
npm i html-webpack-plugin -S
npm i clean-webpack-plugin -S
html-webpack-plugin
//在dist目录下自动生成html,让打包的js文件自动插入
const HtmlWebpackPlugin = require('html-webpack-plugin');
plugins: [
...
new HtmlWebpackPlugin({
title: "webpack测试"
})
]
clean-webpack-plugin
//每次打包之前会将dist目录下的文件清除
const { CleanWebpackPlugin } = require('clean-webpack-plugin');
plugins: [
new CleanWebpackPlugin()
]
webpack-server
//写代码的时候就可以看到效果
npm i webpack-dev-server -S
npm i cross-env -S
(1)配置package.json
"scripts": {
"build": "cross-env NODE_ENV=production webpack --config webpack.config.js",
"serve":"cross-env NODE_ENV=development webpack-dev-server --config webpack.config.js"
}
(2)配置webpack.config.js
const isDev = process.env.NODE_ENV == 'development'
if (isDev) {
config.devServer = {
host: 'localhost',
port: 8080,
/* 错误是否显示在界面上 */
overlay: {
errors: true
},
/* 是否进行热更新 */
hot: true
}
}
(3)运行
npm run serve
loader
//处理.vue .css .png|jpg
npm i css-loader style-loader -S
npm i vue-loader vue vue-template-compiler -S
npm i file-loader -S
(1)配置webpack.config.js
const {VueLoaderPlugin} = require('vue-loader');
const config = {
plugins:[
new VueLoaderPlugin()
],
module:{
rules:[
{
test:/\.css$/,
use:[
'style-loader',
'css-loader'
]
},
{
test:/\.vue$/,
use:'vue-loader'
},
{
test:/\.png|jpg|gif$/i,
use:'file-loader'
}
]
},
mode:"development"
}
(2)配置src/index.js
import Vue from 'vue'
import '../assets/index.css';
import App from './App.vue';
const root = document.createElement("div");
document.body.append(root)
new Vue({
render:h=>h(App)
}).$mount(root)
(3)运行
npm run serve //查看效果
求网络数据
npm i axios -S
<script>
import axios from 'axios'
export default {
name: "App",
data(){
return {
musics:[]
}
},
mounted() {
var url = "http://192.168.1.19:3000/top/playlist?cat=appblog";
// this.axios(url).then(res=>{
// console.log(res)
// })
axios.get(url).then(res=>{
this.musics = res.data.playlists
})
}
};
</script>
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/12/webpack-core-concepts-and-introduction/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

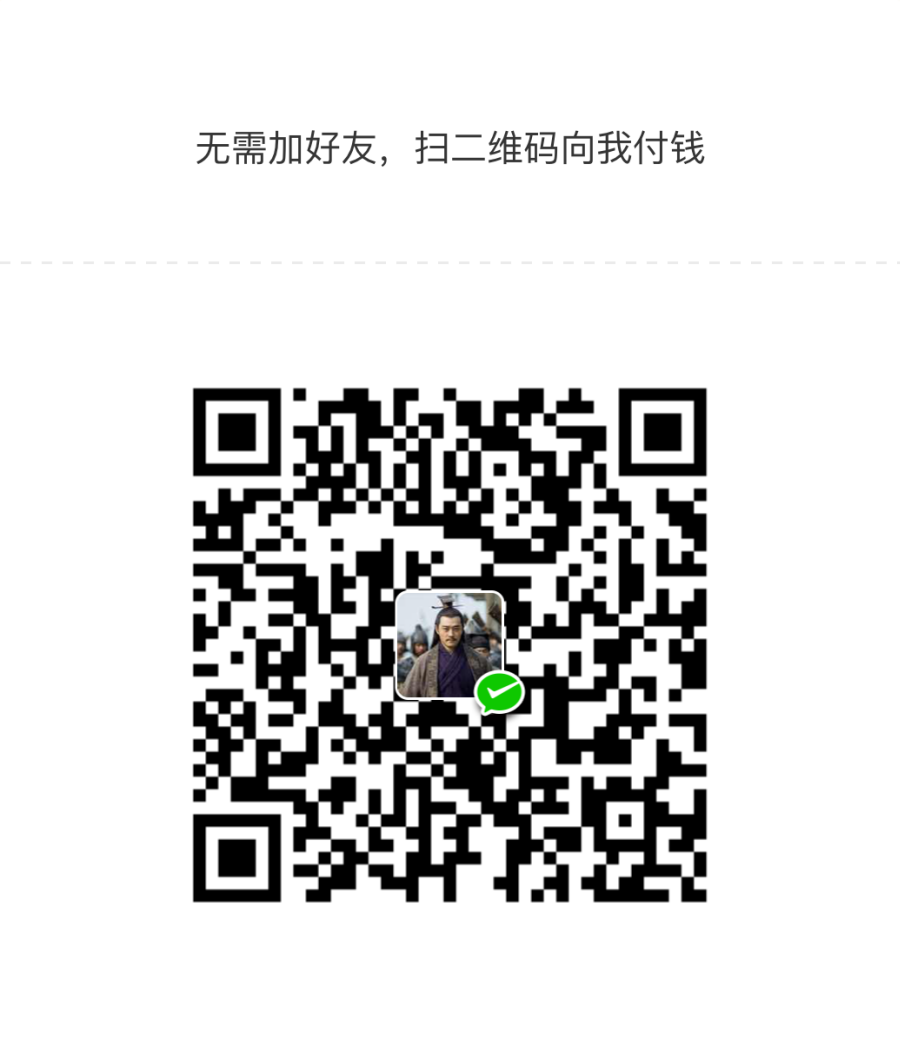

共有 0 条评论