Spring Security OAuth2 JWT 资源服务器配置
POM相关依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.6.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-jwt</artifactId>
<version>1.0.10.RELEASE</version>
</dependency>
添加资源服务器配置
@EnableResourceServer
注解实际上相当于在拦截器链之中帮我们加上了OAuth2AuthenticationProcessingFilter
过滤器,拦截器会拦截参数中的access_token
及Header
头中是否
添加有Authorization
,并且Authorization
是以Bearer
开头的access_token
才能够识别;过滤器中相关的接口有TokenExtractor
,其实现类是BearerTokenExtractor
。
package cn.appblog.security.resource.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configurers.ResourceServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.DefaultTokenServices;
import org.springframework.security.oauth2.provider.token.TokenStore;
import org.springframework.security.oauth2.provider.token.store.JwtAccessTokenConverter;
import org.springframework.security.oauth2.provider.token.store.JwtTokenStore;
/**
* @Description: @EnableResourceServer注解实际上相当于加上OAuth2AuthenticationProcessingFilter过滤器
* @Package: cn.appblog.security.oauth2.config.ResServerConfig
* @Version: 1.0
*/
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources
.tokenServices(tokenServices())
//资源ID
.resourceId("resource_password_id");
super.configure(resources);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest()
.permitAll();
http.csrf().disable();
}
/**
* OAuth2 token持久化接口,jwt不会做持久化处理
*/
@Bean
public TokenStore jwtTokenStore() {
return new JwtTokenStore(accessTokenConverter());
}
/**
* 令牌服务
*/
@Bean
public DefaultTokenServices tokenServices() {
DefaultTokenServices defaultTokenServices = new DefaultTokenServices();
defaultTokenServices.setTokenStore(jwtTokenStore());
return defaultTokenServices;
}
/**
* 自定义token令牌增强器
*/
@Bean
public JwtAccessTokenConverter accessTokenConverter() {
JwtAccessTokenConverter accessTokenConverter = new JwtAccessTokenConverter();
accessTokenConverter.setSigningKey("123456");
return accessTokenConverter;
}
/**
* 加密方式
*/
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
新增资源服务接口
package cn.appblog.security.resource.api;
import org.springframework.security.core.context.SecurityContext;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
/**
* @Description: 资源服务器
* @Package: ResourceController
* @Version: 1.0
*/
@RestController
@RequestMapping("/resource")
public class ResourceController {
@RequestMapping(value = "context", method = RequestMethod.GET)
@ResponseBody
public Object get() {
SecurityContext ctx = SecurityContextHolder.getContext();
return ctx;
}
}
启动服务类
@SpringBootApplication(scanBasePackages = {"cn.appblog.security.resource"})
public class ResourceJwtApplication {
public static void main(String[] args) {
SpringApplication.run(ResourceJwtApplication.class, args);
}
}
访问测试
(1)访问:http://127.0.0.1:9002/resource/context
{
"authentication":{
"authorities":[
{
"authority":"ROLE_ANONYMOUS"
}
],
"details":{
"remoteAddress":"127.0.0.1",
"sessionId":null
},
"authenticated":true,
"principal":"anonymousUser",
"keyHash":-872685687,
"credentials":"",
"name":"anonymousUser"
}
}
{
"authentication":{
"authorities":[
{
"authority":"ROLE"
}
],
"details":{
"remoteAddress":"127.0.0.1",
"sessionId":null,
"tokenValue":"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJwcmluY2lwYWwiOlt7ImF1dGhvcml0eSI6IlJPTEUifV0sImF1ZCI6WyJyZXNvdXJjZV9wYXNzd29yZF9pZCJdLCJ1c2VyX25hbWUiOiJ1c2VyIiwic2NvcGUiOlsidGVzdCIsImNlc2hpIl0sImV4cCI6MTU2NTc2NDYyMywiYXV0aG9yaXRpZXMiOlsiUk9MRSJdLCJqdGkiOiIwMWQ0M2I1NS1iNzlkLTRmMjUtYTllYS0xNGQxZWQxZTQ4YzMiLCJjbGllbnRfaWQiOiJjbGllbnRfcGFzc3dvcmQiLCJ1c2VybmFtZSI6InVzZXIifQ.xEciTuJEvTNqhvyQqwvkKhk9w5EPwuQFYfpB1A2hZEY",
"tokenType":"Bearer",
"decodedDetails":null
},
"authenticated":true,
"userAuthentication":{
"authorities":[
{
"authority":"ROLE"
}
],
"details":null,
"authenticated":true,
"principal":"user",
"credentials":"N/A",
"name":"user"
},
"oauth2Request":{
"clientId":"client_password",
"scope":[
"test",
"ceshi"
],
"requestParameters":{
"client_id":"client_password"
},
"resourceIds":[
"resource_password_id"
],
"authorities":[
],
"approved":true,
"refresh":false,
"redirectUri":null,
"responseTypes":[
],
"extensions":{
},
"refreshTokenRequest":null,
"grantType":null
},
"clientOnly":false,
"principal":"user",
"credentials":"",
"name":"user"
}
}
本文转载参考 原文 并加以调试
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/19/spring-security-oauth2-jwt-resource-server-configuration/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

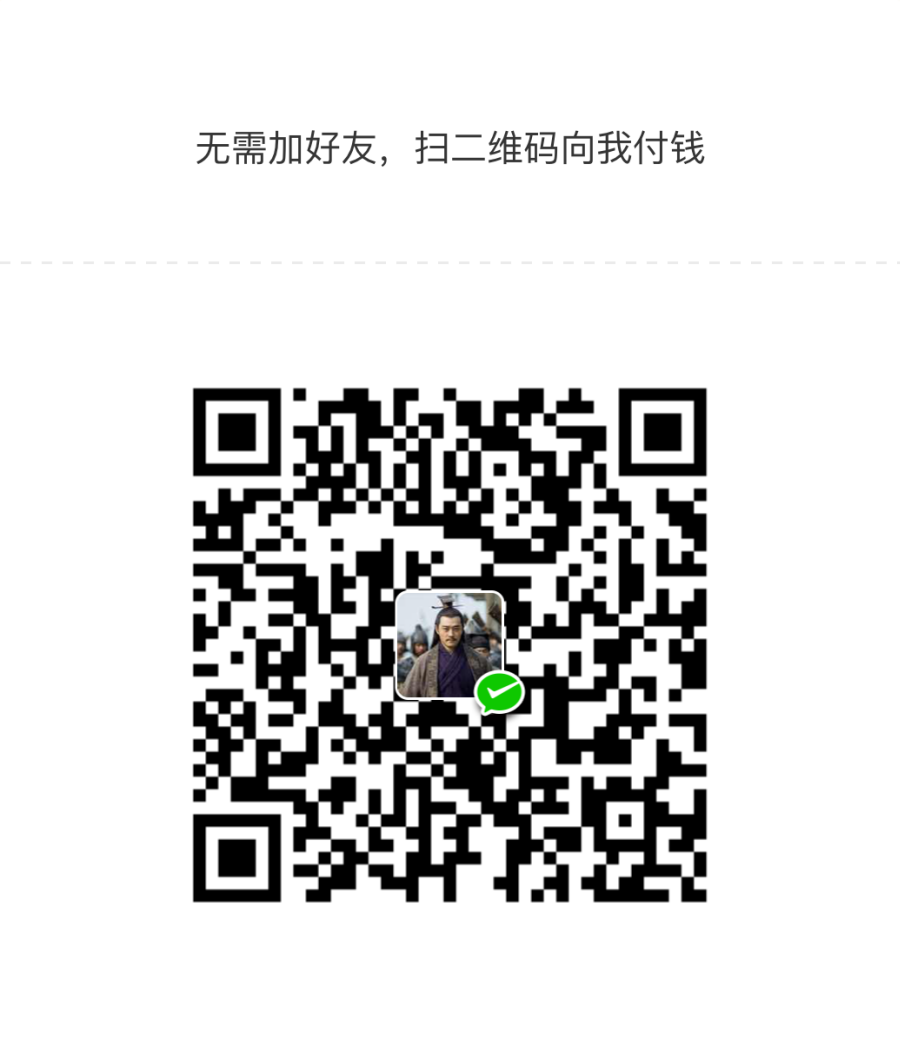
Spring Security OAuth2 JWT 资源服务器配置
POM相关依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</ar……

文章目录
关闭
共有 0 条评论