Spring Security OAuth2 password模式、refresh_token模式访问/oauth/token端点
/oauth/token 端点
- 端点过滤器
TokenEndpointAuthenticationFilter
- 端点对应的action类
TokenEndpoint
- 受保护的资源信息类
ResourceOwnerPasswordResourceDetails
- 和认证服务器交互资源信息类
ResourceOwnerPasswordAccessTokenProvider
/oauth/token(令牌端点)获取用户token信息
/**
* /oauth/token(令牌端点) 获取用户token信息
*/
@RequestMapping(value = "token", method = RequestMethod.POST)
public ResponseEntity<BaseResponse> getToken(@RequestParam String username, @RequestParam String password) {
ResourceOwnerPasswordResourceDetails resource = new ResourceOwnerPasswordResourceDetails();
resource.setId(propertyService.getProperty("spring.security.oauth.resource.id"));
resource.setClientId(propertyService.getProperty("spring.security.oauth.resource.client.id"));
resource.setClientSecret(propertyService.getProperty("spring.security.oauth.resource.client.secret"));
resource.setGrantType(GrantTypeEnum.PASSWORD.getGrant_type());
resource.setAccessTokenUri(propertyService.getProperty("spring.security.oauth.token.uri"));
resource.setUsername(username);
resource.setPassword(password);
resource.setScope(Arrays.asList("all"));
OAuth2RestTemplate template = new OAuth2RestTemplate(resource);
ResourceOwnerPasswordAccessTokenProvider provider = new ResourceOwnerPasswordAccessTokenProvider();
template.setAccessTokenProvider(provider);
try {
OAuth2AccessToken accessToken = template.getAccessToken();
Map<String, Object> result = Maps.newHashMap();
result.put("access_token", accessToken.getValue());
result.put("token_type", accessToken.getTokenType());
result.put("refresh_token", accessToken.getRefreshToken().getValue());
result.put("expires_in", accessToken.getExpiresIn());
result.put("scope", StringUtils.join(accessToken.getScope(), ","));
result.putAll(accessToken.getAdditionalInformation());
Collection<? extends GrantedAuthority> authorities = tokenStore.readAuthentication(template.getAccessToken()).getUserAuthentication().getAuthorities();
JSONObject jsonObject = new JSONObject();
for (GrantedAuthority authority : authorities) {
jsonObject.putAll(JSONObject.parseObject(authority.getAuthority()));
}
result.put("authorities", jsonObject);
return ResponseEntity.ok(BaseResponse.createResponse(HttpStatusMsg.OK, result));
} catch (Exception e) {
e.printStackTrace();
return ResponseEntity.ok(BaseResponse.createResponse(HttpStatusMsg.AUTHENTICATION_EXCEPTION, e.toString()));
}
}
POST访问:http://127.0.0.1:9003/oauth2/token
参数:username、password
{
"status": 200,
"message": "SUCCESS",
"data": {
"access_token": "574007641a804ebf871248991e20bec6",
"refresh_token": "b250860394954b2ea47b7f40563a027d",
"scope": "all",
"token_type": "bearer",
"expires_in": 59,
"client_id": "client_password",
"authorities": {
"interfaces": [
"/a/b",
"/a/c",
"/oauth/token"
],
"username": "admin"
}
}
}
/oauth/token(令牌端点)刷新token信息
/**
* /oauth/token(令牌端点)刷新token信息
*/
@RequestMapping(value = "refresh_token", method = RequestMethod.POST)
public ResponseEntity<BaseResponse> refreshToken(@RequestParam String refresh_token) {
try {
ResourceOwnerPasswordResourceDetails resource = new ResourceOwnerPasswordResourceDetails();
resource.setId(propertyService.getProperty("spring.security.oauth.resource.id"));
resource.setClientId(propertyService.getProperty("spring.security.oauth.resource.client.id"));
resource.setClientSecret(propertyService.getProperty("spring.security.oauth.resource.client.secret"));
resource.setGrantType(GrantTypeEnum.REFRESH_TOKEN.getGrant_type());
resource.setAccessTokenUri(propertyService.getProperty("spring.security.oauth.token.uri"));
ResourceOwnerPasswordAccessTokenProvider provider = new ResourceOwnerPasswordAccessTokenProvider();
OAuth2RefreshToken refreshToken = tokenStore.readRefreshToken(refresh_token);
OAuth2AccessToken accessToken = provider.refreshAccessToken(resource, refreshToken, new DefaultAccessTokenRequest());
Map<String, Object> result = Maps.newLinkedHashMap();
result.put("access_token", accessToken.getValue());
result.put("token_type", accessToken.getTokenType());
result.put("refresh_token", accessToken.getRefreshToken().getValue());
result.put("expires_in", accessToken.getExpiresIn());
result.put("scope", StringUtils.join(accessToken.getScope(), ","));
result.putAll(accessToken.getAdditionalInformation());
Collection<? extends GrantedAuthority> authorities = tokenStore.readAuthentication(accessToken).getUserAuthentication().getAuthorities();
JSONObject jsonObject = new JSONObject();
for (GrantedAuthority authority : authorities) {
jsonObject.putAll(JSONObject.parseObject(authority.getAuthority()));
}
result.put("authorities", jsonObject);
return ResponseEntity.ok(BaseResponse.createResponse(HttpStatusMsg.OK, result));
} catch (Exception e) {
e.printStackTrace();
return ResponseEntity.ok(BaseResponse.createResponse(HttpStatusMsg.AUTHENTICATION_EXCEPTION, e.toString()));
}
}
POST访问:http://127.0.0.1:9003/oauth2/refresh_token
参数:refresh_token
{
"status": 200,
"message": "SUCCESS",
"data": {
"access_token": "b4b26e5aab854b3aa44b4983901fd7ac",
"token_type": "bearer",
"refresh_token": "dc03d64f523048f9bce62068b1100a4d",
"expires_in": 59,
"scope": "all",
"client_id": "client_password",
"authorities": {
"interfaces": [
"/a/b",
"/a/c",
"/oauth/token"
],
"username": "admin"
}
}
}
oauth/check_token(端点校验)校验token有效性
/**
* oauth/check_token(端点校验)校验token有效性
*/
@RequestMapping(value = "check_token", method = RequestMethod.POST)
public ResponseEntity<BaseResponse> checkToken(String access_token) {
OAuth2AccessToken accessToken = tokenStore.readAccessToken(access_token);
OAuth2Authentication auth2Authentication = tokenStore.readAuthentication(access_token);
Map<String, Object> map = Maps.newHashMap();
//用户名
map.put("username", auth2Authentication.getUserAuthentication().getName());
//是否过期
map.put("isExpired", accessToken.isExpired());
//过期时间
map.put("expiration", DateFormatUtils.format(accessToken.getExpiration(), "yyyy-MM-dd HH:mm:ss"));
BaseResponse response = null;
try {
response = BaseResponse.createResponse(HttpStatusMsg.OK, map);
} catch (Exception e) {
response = BaseResponse.createResponse(HttpStatusMsg.AUTHENTICATION_EXCEPTION, e.toString());
}
return ResponseEntity.ok(response);
}
POST访问:http://127.0.0.1:9003/oauth2/check_token
参数:access_token
{
"status": 200,
"message": "SUCCESS",
"data": {
"expiration": "2019-08-14 16:45:59",
"isExpired": false,
"username": "admin"
}
}
本文转载参考 原文 并加以调试
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/20/spring-security-oauth2-password-mode-refresh-token-mode-access-auth-token-endpoint/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

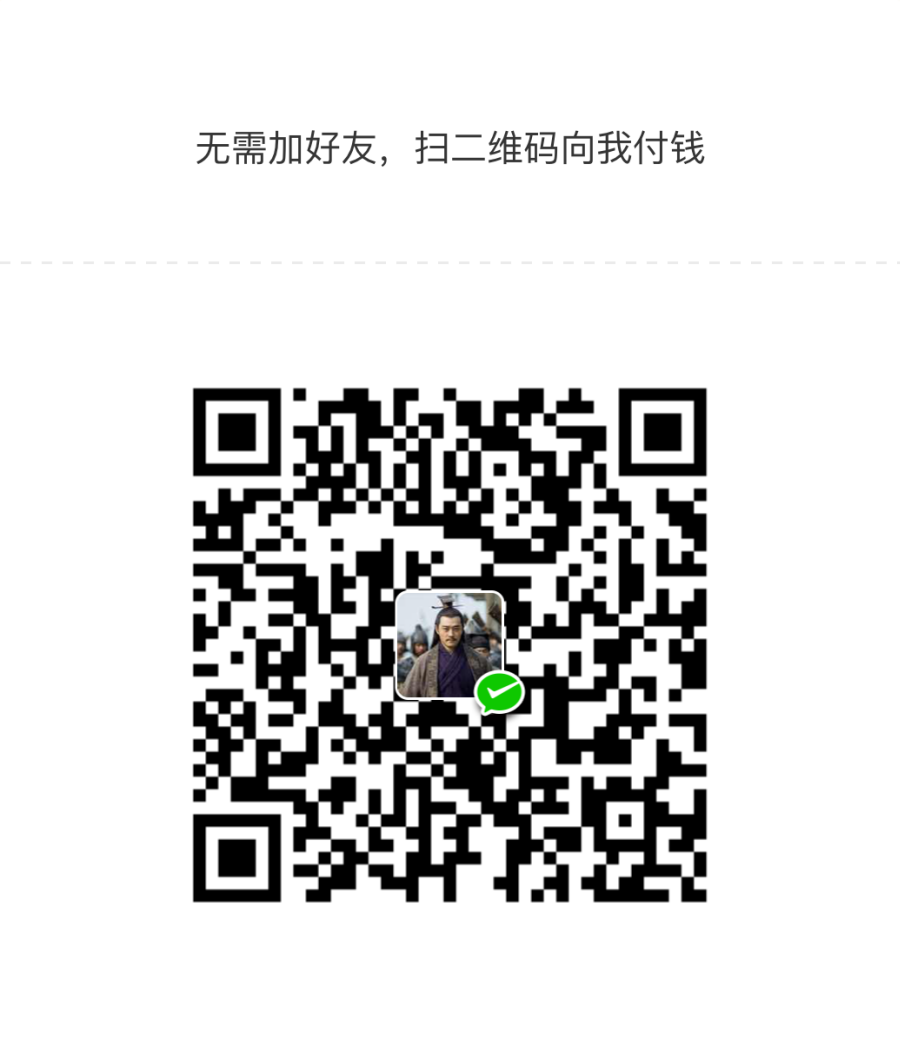
Spring Security OAuth2 password模式、refresh_token模式访问/oauth/token端点
/oauth/token 端点
端点过滤器TokenEndpointAuthenticationFilter
端点对应的action类TokenEndpoint
受保护的资源信息类ResourceOwnerPasswordResourceDetail……

文章目录
关闭
共有 0 条评论