企业微信Java API接入
相关资源
入门文档:https://work.weixin.qq.com/api/doc#90000/90003/90487
服务端API:https://work.weixin.qq.com/api/doc#90000/90135/90664
客户端API:https://work.weixin.qq.com/api/doc#90000/90136/90289
开发者工具:https://work.weixin.qq.com/api/doc#90000/90138/90678
接口调试工具:https://work.weixin.qq.com/api/devtools/devtool.php
WxJava - 微信开发 Java SDK(开发工具包):https://github.com/Wechat-Group/WxJava
群机器人:https://work.weixin.qq.com/help?person_id=1&doc_id=13376
基本配置
<xml>
<corpId>xxx</corpId>
<corpSecret>xxx</corpSecret>
<agentId>1000002</agentId>
<userId>@all</userId>
<departmentId>1</departmentId>
</xml>
@Slf4j
@Component
public class WxCpConfig {
private static final String TEST_CONFIG_XML = "wx-cp.config.xml";
private WxCpService wxService;
private WxXmlCpInMemoryConfigStorage configStorage;
private static <T> T fromXml(Class<T> clazz, InputStream is) {
XStream xstream = XStreamInitializer.getInstance();
xstream.addPermission(AnyTypePermission.ANY);
xstream.alias("xml", clazz);
xstream.processAnnotations(clazz);
return (T) xstream.fromXML(is);
}
@PostConstruct
public void init() {
try (InputStream inputStream = ClassLoader.getSystemResourceAsStream(TEST_CONFIG_XML)) {
if (inputStream == null) {
throw new RuntimeException("测试配置文件【" + TEST_CONFIG_XML + "】未找到,请参照test-config-sample.xml文件生成");
}
configStorage = fromXml(WxXmlCpInMemoryConfigStorage.class, inputStream);
wxService = new WxCpServiceImpl();
wxService.setWxCpConfigStorage(configStorage);
} catch (IOException e) {
log.error(e.getMessage(), e);
}
}
@XStreamAlias("xml")
public static class WxXmlCpInMemoryConfigStorage extends WxCpDefaultConfigImpl {
protected String userId;
protected String departmentId;
protected String tagId;
protected String externalUserId;
public String getUserId() {
return this.userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getDepartmentId() {
return this.departmentId;
}
public void setDepartmentId(String departmentId) {
this.departmentId = departmentId;
}
public String getTagId() {
return this.tagId;
}
public void setTagId(String tagId) {
this.tagId = tagId;
}
public String getExternalUserId() {
return externalUserId;
}
public void setExternalUserId(String externalUserId) {
this.externalUserId = externalUserId;
}
@Override
public String toString() {
return super.toString() + " > WxXmlCpConfigStorage{" +
"userId='" + this.userId + '\'' +
", departmentId='" + this.departmentId + '\'' +
", tagId='" + this.tagId + '\'' +
", externalUserId='" + this.externalUserId + '\'' +
'}';
}
}
public WxCpService getWxService() {
return wxService;
}
}
API调用
@Slf4j
@Service
public class WxCpCoreService {
@Autowired
public WxCpConfig wxCpConfig;
@SuppressWarnings("unchecked")
public WxCpResponse sendMessage(WxCpRequest wxCpRequest) {
try {
WxCpService wxCpService = wxCpConfig.getWxService();
WxCpConfig.WxXmlCpInMemoryConfigStorage configStorage = (WxCpConfig.WxXmlCpInMemoryConfigStorage) wxCpService.getWxCpConfigStorage();
String before = configStorage.getAccessToken();
log.info("before: " + before);
wxCpService.getAccessToken(false);
String after = configStorage.getAccessToken();
log.info("after: " + after);
WxCpDepartmentService wxCpDepartmentService = wxCpService.getDepartmentService();
List<WxCpDepart> departList = wxCpDepartmentService.list(1L);
if (departList != null && departList.size() > 0) {
for (int i = 0; i < departList.size(); i++) {
log.info("Depart id: {}", departList.get(i).getId());
}
}
WxCpUserService wxCpUserService = wxCpService.getUserService();
List<WxCpUser> userList = wxCpUserService.listByDepartment(1L, true, 0);
if (userList != null && userList.size() > 0) {
for (int i = 0; i < userList.size(); i++) {
log.info("User id: {}", userList.get(i).getUserId());
}
}
// WxCpMessage message = WxCpMessage
// .TEXT()
// .toParty(configStorage.getDepartmentId())
// .toUser(configStorage.getUserId())
// .content("欢迎欢迎,热烈欢迎\n换行测试\n超链接:<a href=\"http://www.appblog.cn\">Hello World</a>")
// .build();
WxCpMessage message = new WxCpMessage();
message.setMsgType(WxConsts.KefuMsgType.MARKDOWN);
message.setToParty(configStorage.getDepartmentId());
message.setToUser(configStorage.getUserId());
message.setContent("您的会议室已经预定,稍后会同步到`邮箱` \n" +
" >**事项详情** \n" +
" >事 项:<font color=\\\"info\\\">开会</font> \n" +
" >组织者:@Joe.Ye \n" +
" >参与者:@Joe.Ye、@AppBlog.CN、@China、@HangZhou、@ShangHai \n" +
" > \n" +
" >会议室:<font color=\\\"info\\\">杭州国际会议中心 1楼 101</font> \n" +
" >日 期:<font color=\\\"warning\\\">2019年11月30日</font> \n" +
" >时 间:<font color=\\\"comment\\\">上午9:00-11:00</font> \n" +
" > \n" +
" >请准时参加会议。 \n" +
" > \n" +
" >如需修改会议信息,请点击:[修改会议信息](https://work.weixin.qq.com)");
WxCpMessageSendResult messageSendResult = wxCpService.messageSend(message);
log.info("message: {}", JSON.toJSONString(message));
log.info("WxCpCoreService.sendMessage messageSendResult: " + JSON.toJSONString(messageSendResult));
return new WxCpResponse();
} catch (Exception e) {
log.error("WxCpCoreService.sendMessage exception: ", e);
return null;
}
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/26/enterprise-wechat-java-api-access/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

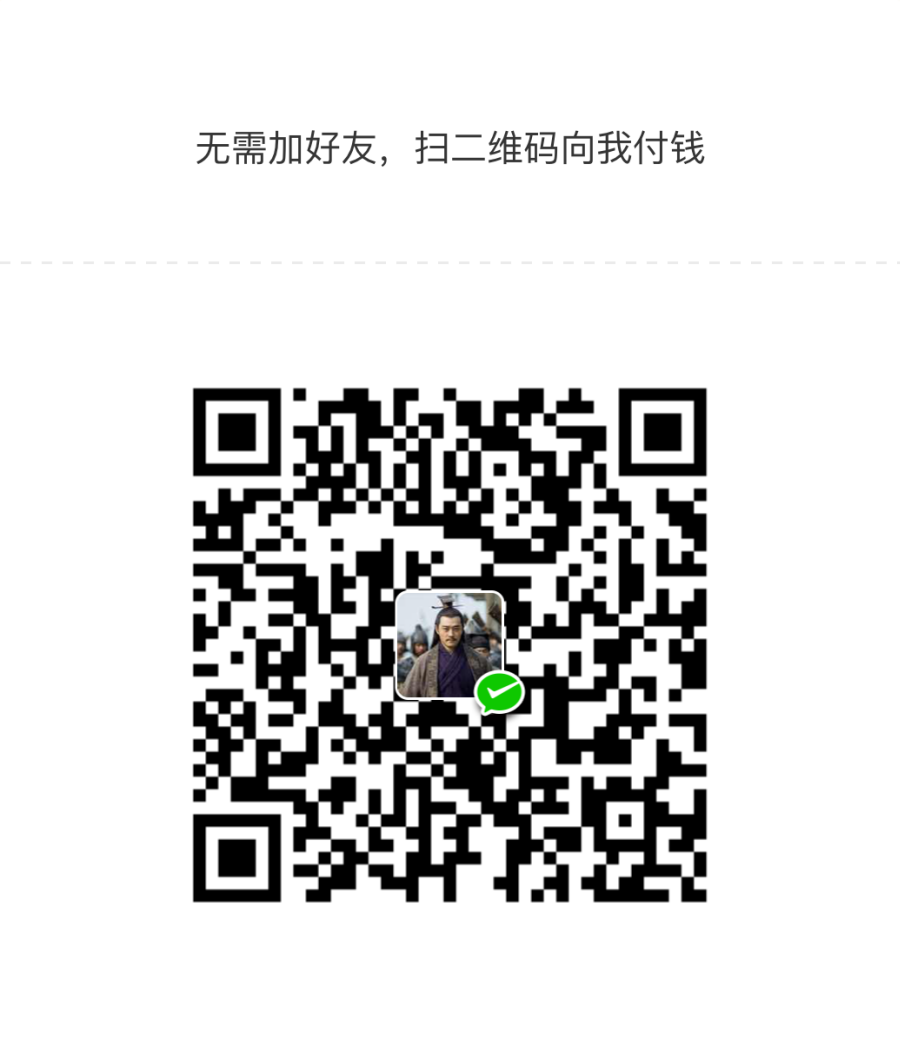

共有 0 条评论