Spring Boot四大组件
Spring Boot特点
- 遵循约定优于配置的原则
- 提供starter pom,高效包管理
- 简化配置,无需xml
- 内嵌Servlet容器
- 与主流框架集成简单
Spring Boot四大组件
Auto-Configuration
运行依赖:
- 继承
spring-boot-starter-parent
- 继承
spring-boot-dependencies
- 引入
spring-boot-autoconfigure
运行机制:
- 注解
EnableAutoConfiguration
AutoConfigurationImportSelector
- 通过
selectImports
加载自动配置信息 - 通过
getCandidateConfigurations
加载META-INF/spring.factories
官方集成框架
- RabbitAutoConfiguration
- JpaRepositoriesAutoConfiguration
- ElasticSearchAutoConfiguration
- RedisAutoConfiguration
- LdapDataAutoConfiguration
- MongoAutoConfiguration
第三方集成框架
- MybatisAutoConfiguration
- DubboAutoConfiguration
- PageHelperAutoConfiguration
常用注解
@Configuration
:Spring上下文配置@Bean
:对象注解@ConditionalOnBean
:当SpringIoc容器内存在指定Bean的条件@ConditionalOnClass
:当SpringIoc容器内存在指定Class的条件@ConditionalOnMissingBean
:当SpringIoc容器内不存在指定Bean的条件@ConditionalOnMissingClass
:当SpringIoc容器内不存在指定Class的条件@ConditionalOnProperty
:指定的属性是否有指定的值@ConditionalOnResource
:类路径是否有指定的值
Starter
Starter是Spring Boot中的一个非常重要的概念,Starter相当于模块,它能将模块所需的依赖整合起来并对模块内的Bean根据环境(条件)进行自动配置。使用者只需要依赖相应功能的Starter,无需做过多的配置和依赖,Spring Boot就能自动扫描并加载相应的模块。
常用Starter
- The Web Starter
- The Data JPA Starter
- mybatis-spring-boot-starter
- pagehelper-spring-boot-starter
- dubbo-spring-boot-starter
- The Mail Starter
- The Test Starter
开发Starter步骤
- 新建Maven项目,在项目的pom文件中定义使用的依赖
- 新建配置类,写好配置项和默认的配置值,指明配置项前缀
- 新建自动装配类,使用
@Configuration
和@Bean
来进行自动装配 - 新建spring.factories文件,指定Starter的自动装配类
Starter实例
(1)定义开关注解
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Enable AppBlog DevTool for spring boot application
*/
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface EnableAppBlogConfiguration {
}
(2)定义约定配置属性
@ConfigurationProperties(prefix = "spring.appblog")
public class AppBlogProperties {
private String name = null;
}
(3)定义自动配置类
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import cn.appblog.annotation.EnableAppBlogConfiguration;
@Configuration
@ConditionalOnBean(annotation = EnableAppBlogConfiguration.class)
@EnableConfigurationProperties(AppBlogProperties.class)
public class AppBlogConfiguration {
@Autowired
private AppBlogProperties properties;
@Bean
public Object createBean() {
System.out.println("AppBlog=" + properties);
return new Object();
}
}
(4)定义启动Banner
package cn.appblog.boot.context.event;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.context.event.ApplicationEnvironmentPreparedEvent;
import org.springframework.context.ApplicationListener;
import cn.appblog.annotation.EnableAppBlogConfiguration;
@ConditionalOnBean(annotation = EnableAppBlogConfiguration.class)
public class AppBlogBannerApplicationListener implements
ApplicationListener<ApplicationEnvironmentPreparedEvent>
{
public static String appblogLogo =
" ################################################################################## \n" +
" ######## _ ____ _ ____ _ _ ######## \n" +
" ######## / \ _ __ _ __ | __ )| | ___ __ _ / ___| \ | | ######## \n" +
" ######## / _ \ | '_ \| '_ \| _ \| |/ _ \ / _` || | | \| | ######## \n" +
" ######## / ___ \| |_) | |_) | |_) | | (_) | (_| || |___| |\ | ######## \n" +
" ######## /_/ \_\ .__/| .__/|____/|_|\___/ \__, (_)____|_| \_| ######## \n" +
" ######## |_| |_| |___/ ######## \n" +
" ######## ######## \n" +
" ################################################################################## \n" +
" \n" +
"\n";
public static String LINE_SEPARATOR = System.getProperty("line.separator");
@Override
public void onApplicationEvent(ApplicationEnvironmentPreparedEvent event) {
System.out.println(buildBannerText());
}
private String buildBannerText() {
StringBuilder bannerTextBuilder = new StringBuilder();
bannerTextBuilder.append(LINE_SEPARATOR).append(appblogLogo).append(" :: AppBlog :: (v1.0.0)")
.append(LINE_SEPARATOR);
return bannerTextBuilder.toString();
}
}
(5)定义Starter配置文件
resources/META-INF/spring.factories
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
cn.appblog.boot.AppBlogConfiguration
org.springframework.context.ApplicationListener=\
cn.appblog.boot.context.event.AppBlogBannerApplicationListener
resources/META-INF/spring.provides
provides: appblog-spring-boot-starter
(5)安装到本地Maven仓库
maven install
(6)使用自定义Starter
<dependency>
<groupId>cn.appblog</groupId>
<artifactId>appblog-spring-boot-starter</artifactId>
<version>1.0.0-SNAPSHOT</version>
</dependency>
//SpringBoot启动排除RabbitMQ自动配置
@SpringBootApplication(exclude={RabbitAutoConfiguration.class})
@EnableAppBlogConfiguration
public class StarterApplication {
public static void main(String[] args) {
SpringApplication.run(StarterApplication.class, args);
}
}
Spring Boot Cli
Spring Boot Cli安装
https://repo.spring.io/release/org/springframework/boot/spring-boot-cli
https://repo.spring.io/release/org/springframework/boot/spring-boot-cli/2.3.3.RELEASE/spring-boot-cli-2.3.3.RELEASE-bin.tar.gz
下载解压并设置环境变量path
spring --version
spring help //获取帮助
spring help <command> //查询某个命令的帮助
spring init <options> //命令参数
spring init --list //列出服务内容
使用实例
(1)创建支持 web,data-rest,jpa,jdbc,jooq,mail 的Spring Boot工程
spring init -dweb,data-rest,jpa,jdbc,jooq,mail demo
(2)创建Maven工程
spring init --build maven -j 1.8 -dweb,jpa,security -b 2.2.3.RELEASE -g cn.appblog -p jar myapp -a hello-boot -v 1.0.0-SNAPSHOT
使用命令启动项目
mvn spring-boot:run
使用 Spring Boot Cli 运行单独 Groovy 脚本服务
(1)创建 hello.groovy
@RestController
public class HelloController {
@RequestMapping(value = "/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
(2)使用 Spring Boot Cli 运行
spring run hello.groovy
使用 Spring Boot Cli 运行单独 Java 脚本服务
(1)创建 Hello.java
@RestController
public class HelloController {
@RequestMapping(value = "/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
(2)使用 Spring Boot Cli 运行
spring run Hello.java
Actuator
Actuator是Spring Boot提供的对应用系统的自身和监控的集成功能,可以对应用系统进行配置查看、相关功能统计。
Spring Boot集成Actuator,在pom.xml中添加Actuator依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<groupId>spring-boot-starter-actuator</groupId>
</dependency>
配置信息
# actuator监控端口
management.server.port=8100
management.server.servlet.context-path=/actuator
# 默认开启health和info,设置*则包括所有web端口
management.endpoints.web.exposure.include=*
# 打开默认关闭的shutdown端点
management.endpoint.shutdown.enabled=true
通过http协议访问查看内部状态
beans
:显示一个应用中所有Spring Beans的完整列表env
:显示来自Spring的ConfigurationEnvironment
的属性info
:显示任意的应用信息metrics
:展示当前应用的metrics信息mappings
:显示一个所有@RequestMapping
路径的集合列表
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/26/four-major-components-of-spring-boot/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

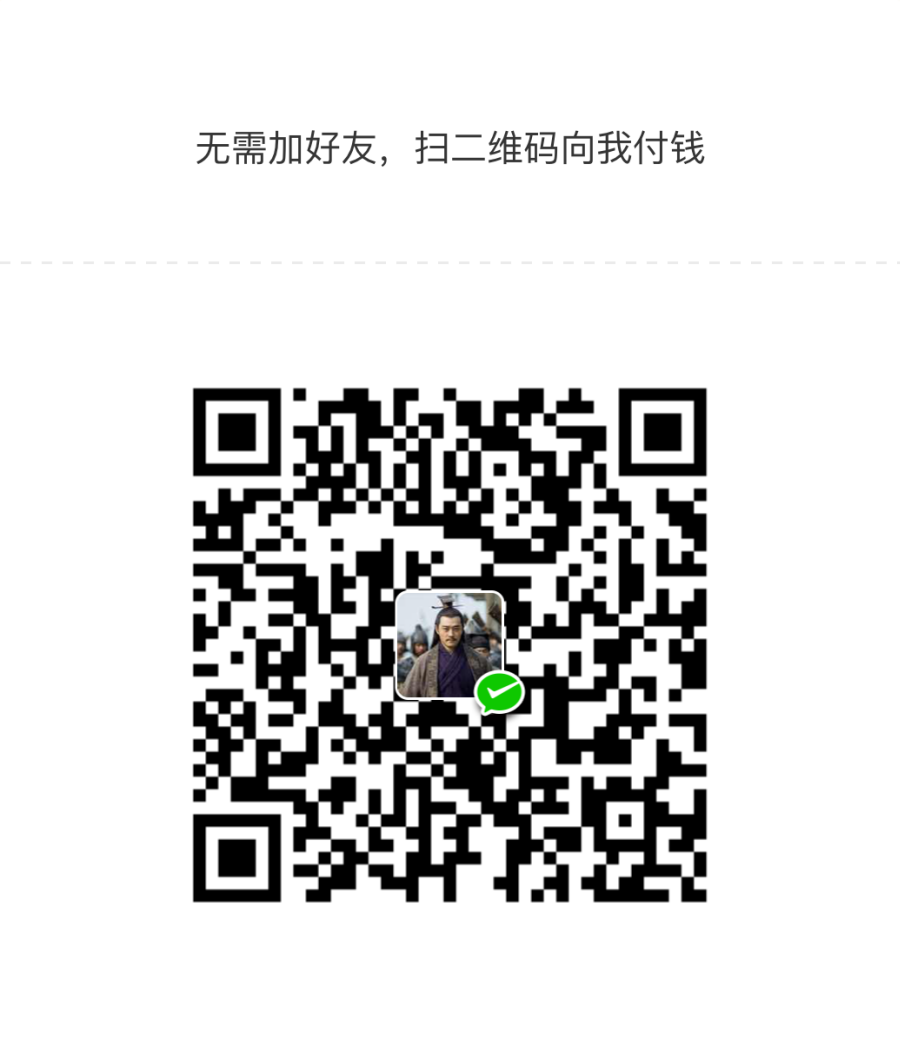

共有 0 条评论