编写自己的Spring Boot Starter
基本步骤
- 引入对应的依赖
- 编写实现类
- 编写配置文件读取类,主要注解是
@ConfigurationProperties
(配置的值例如a.b
) - 编写自动装配类
- 编写默认的配置文件
resources/META-INF/spring.factories
在resources/META-INF/spring.factories
中配置我们的自动装配类
具体编码
引入的依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
</dependency>
</dependencies>
说明:
- 第一个依赖 主要是为编译器配置的,可以根据
yml
或properties
配置项鼠标右键,点到使用这个属性的类上 - 第二个依赖 主要是为了自动装配
编写自己的功能实现类
为了说明问题 实现类的作用就是返回配置字符串的hashcode
package cn.appblog;
/**
* 目标功能实现类
*/
public class HashCodeClient {
private String target;
public HashCodeClient(String target){
this.target = target;
}
public String getHashCode() {
return String.valueOf(this.target.hashCode());
}
}
编写配置文件读取类
package cn.appblog;
import org.springframework.boot.context.properties.ConfigurationProperties;
@ConfigurationProperties("cn.appblog")
public class HashCodeProperties {
private String target;
public String getTarget() {
return target;
}
public void setTarget(String target) {
this.target = target;
}
}
这里我们要读取的配置就是cn.appblog.target
的值,@ConfigurationProperties
注解的作用就是读取配置文件指定属性的值
编写自动装配类
package cn.appblog;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableConfigurationProperties(HashCodeProperties.class)
@ConditionalOnClass(HashCodeClient.class)
public class HashCodeAutoConfigrution {
@Autowired
private HashCodeProperties hashCodeProperties;
@ConditionalOnMissingBean
@Bean
public HashCodeClient getHashCodeClient(){
return new HashCodeClient(hashCodeProperties.getTarget());
}
}
@Configuration
标识本类是配置类(相当于spring中application.xml)
@EnableConfigurationProperties(HashCodeProperties.class)
如果HashCodeProperties
中有注解@ConfigurationProperties
那么这个类就会被加到spring上下文的容器中,也就是可以通过@Autowire
来注入
@ConditionalOnClass
当类路径下有指定类的情况下才进行下一步
@ConditionalOnMissingBean
当spring容器中没有这个Bean时才进行下一步
设置自动加载的配置文件spring.factories
在resources/META-INF
下添加spring.factories
指定自动装配的类也叫入口,内容如下:
org.springframework.boot.autoconfigure.EnableAutoConfiguration=
cn.appblog.HashCodeAutoConfigrution
添加我们的默认配置
在application.yml
中添加下面
cn:
appblog:
target: www.appblog.cn
这就有了默认值
发布本地仓库或部署私有仓库
通过maven install
命令发布在本地,然后在其他项目引入这个jar依赖,测试时直接自动注入我们的bean即可
测试是否成功
新建Spring Boot工程,引入依赖
<!--引入自定义的starter-->
<dependency>
<groupId>com.test.springboot</groupId>
<artifactId>myself-spring-boot-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
直接进行单元测试
package cn.appblog.test;
import cn.appblog.HashCodeClient;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringBootConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import javax.annotation.Resource;
import java.util.HashMap;
import java.util.Map;
import static org.junit.Assert.*;
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
public class ControllerTest {
@Autowired
private HashCodeClient hashCodeClient;
@Test
public void testStarter(){
System.out.println(hashCodeClient.getHashCode());
}
}
运行成功获取hashCode
在application.yml
中覆盖原来的属性
cn:
appblog:
target: test
测试得hashCode不一致,证明成功
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/26/write-spring-boot-starter/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

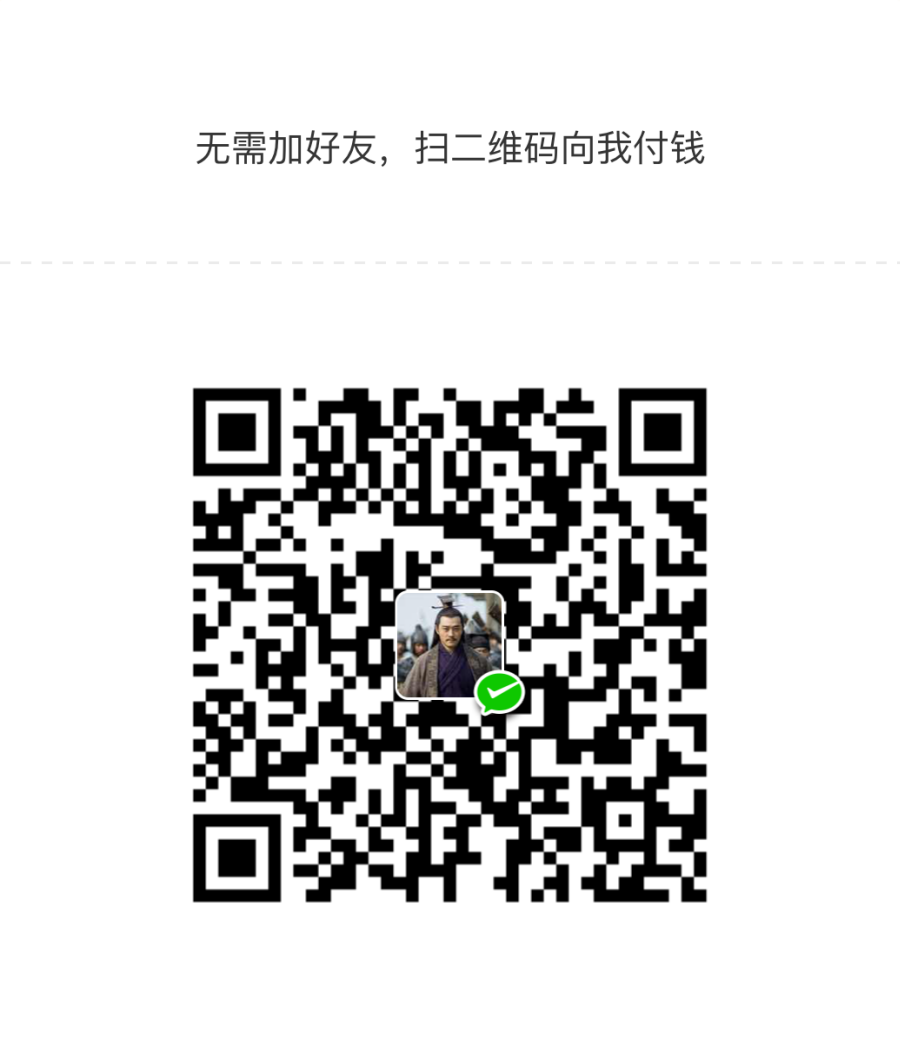

共有 0 条评论